Multiline text on the node text or subitem value.
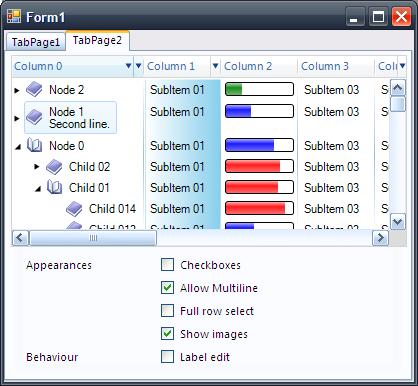
TreeNode Checkboxes. The check box of the tree node doesn't automatically appear when you set the CheckBoxes
property of the MultiColumnTree
object to true
, it will only appear when the tree node is selected, on hover, or the value of the CheckState
property is not unchecked.

The first column is frozen.

The first column is sorted in descending order.

The second column has custom background painting.

Custom filter button on filter popup window.

Column options popup window. It will display columns that have EnableHidden
or EnableFrozen
property set to true
. It allows you to hide or freeze the column on runtime.

Custom tooltip. However, it will be shown if you set the NodeToolTip
property on the MultiColumnTree
object to true
and the tree node has tooltip title, tooltip contents, or tooltip image. If the FullRowSelect
property of the MultiColumnTree
object is set to true
, the tooltip will be displayed when the mouse pointer hovers on the whole node, otherwise, the tooltip will be displayed only when the mouse pointer hovers on the node text.

The appearance when the FullRowSelect
property of the MultiColumnTree
object is set to true
.

Introduction
I need to show additional nodes on a tree, just like what a listview
did. However there is no such control in standard WinForms control. So, I decided to create on my own. A lot of improvement is needed to make this control fully useful.
Changes
I don't use TreeColumn
class anymore. That class has been replaced with ColumnHeader
class because I use it along with my ListView
, and Items
property of the TreeNode
has been replaced with SubItems
property.
Classes
There are classes that correspond with MultiColumnTree
class.
Column (ColumnHeader Class)
Represent a column object for a tree.
- Supports multiple column.
- Supports 4 different types of column size: fixed size, percent, auto, and fill.
- Supports runtime sorting.
- Supports display formatting,
DateTime
, Numeric
, Bar
, and Custom
. However, display format on the column 0 will be ignored. - When
Bar
is used in column format property, you can also specify the minimum and maximum range for the bar. - Supports column filtering, column filtering based on the column format used.
- Supports column background painting. You can paint your custom background of each column here.
- Two different alignments. The alignment of the Text on Column Header depends on
TextAlign
property, and the alignment of the SubItem
value depends on ColumnAlign
property. - Supports tooltip. You can specify the tooltip title, tooltip contents, or tooltip image for each column.
New features of the Column:
- Custom filter. Enable custom filtering operation on each column. This feature will appear if you set
EnableFiltering
and EnableCustomFilter
properties to true
on the ColumnHeader
class. Custom filter will raise ColumnCustomFilter
event on MultiColumnTree
class, and you can set the custom filtering operation to the related column by setting the CustomFilter
property of the ColumnHeader
. Custom filter property has reference type to Delegate Function CustomFilterFunction(ByVal value As Object) As Boolean.
- Enable Frozen and Frozen properties (I don't know how to name this feature). Just like Freeze Pane feature on Microsoft Excel. The Frozen columns will shown on the first order and above on the UnFrozen columns.
- Enable Hidden and Visible properties. Capability to show and hide the column.
Node (TreeNode Class)
Represent a node object for a tree. Below are additional behaviour.
- Supports tooltip title, contents, and image for each node.
- Supports multiline text for node. However, multiline text will appear when the
AllowMultiline
property of the MultiColumnTree
is set to true
. - SubItems property. To set additional information as
SubItem
for the TreeNode
. - One property I adopted from
ListViewItem
, UseNodeStyleForSubItems
property (I changed the name here for node).
SubItem (TreeNode.TreeNodeSubItem Class) :
Represent a SubItem
of a Node
in the MultiColumnTree
. This class has the same behaviours with ListViewItem.ListViewSubItem
class, with several additional features.
- Supports multiline value
PintValueOnBar
property. This property allows you to show the value over the Bar
, when the Column Format is set to Bar
.
Collections
There are 4 collection classes to support MultiColumnTree
class.
TreeNodeCollection
Class. Represent the collection of TreeNode
objects in a MultiColumnTree
control. TreeNode.TreeNodeSubItemCollection
Class. Represent the collection of TreeNode.TreeNodeSubItem
objects in a TreeNode
object. MultiColumnTree.ColumnHeaderCollection
Class. Represent the collection of ColumnHeader
objects in a MultiColumnTree
control. MultiColumnTree.CheckedTreeNodeCollection
Class. Represent the collection on checked TreeNode
objects in a MultiColumnTree
control.
Disadvantages
There are a lot of disadvantages (only the ones I know) compared with standard TreeView
control, and I hope I can cover it, or maybe someone can help to improve this.
- Doesn't support
ImageList
, so, it can't be used to populate a thing like explorer that uses Win32Api calls. - Doesn't support drag and drop operation (this factor makes this control very useless, in my opinion).
Implementation
To use the control, just drag and drop it from toolbox window in your IDE to your form in design view, just like another control.
To add columns to the tree control programmatically, use Columns.Add
method.
Dim aColumn As ColumnHeader = New ColumnHeader
aColumn.Text = "Column 1"
tree.Columns.Add(aColumn)
To add a node to the tree control, use Nodes.Add
method. To add additional items to a node to be shown in the tree control based on its column, use Items.Add
method on a node object.
Dim aNode As TreeNode = New TreeNode
aNode.Text = "Parent Node"
aNode.SubItems.Add("Parent Sub 1")
tree.Nodes.Add(aNode)
To add another node as child node to an existing node, use Nodes.Add
method on a node object.
Dim childNode As TreeNode = New TreeNode
childNode.Text = "Child Node"
childNode.Items.Add("Child Sub 1")
aNode.Nodes.Add(childNode)
Create custom painting on a column(with index 1) background example.
Private Sub tree_BackgroundColumnPaint(ByVal sender As Object, _
ByVal e As ColumnBackgroundPaintEventArgs) Handles tree.BackgroundColumnPaint
If e.ColumnIndex = 1 Then
Dim aBrush As LinearGradientBrush = New LinearGradientBrush_
(e.Rectangle, Color.White, Color.LightBlue, LinearGradientMode.Horizontal)
e.Graphics.FillRectangle(aBrush, e.Rectangle)
aBrush.Dispose()
End If
End Sub
Handling the ColumnCustomFilter
event on MultiColumnTree
object.
Private Sub tree_ColumnCustomFilter(ByVal sender As Object, _
ByVal e As Ai.Control.ColumnCustomFilterEventArgs) Handles tree.ColumnCustomFilter
e.Column.CustomFilter = AddressOf column1CustomFilter
End Sub
Private Function column1CustomFilter(ByVal value As Object) As Boolean
Return True
End Function
There are several bugs I received from others, and (hopefully, because I am working all alone, I can't test this control completely) have been fixed.
- Lost label after editing bug.
- Check box doesn't automatically appears when you set the
CheckBoxes
property to true
, it will appear when the CheckState
of the node is not unchecked, or the mouse pointer is hovering on a node, or the node is selected. - I create my test project using Tab control, a
Button
on page 1, and MultiColumnTree
control on page 2, and I can populate the node in MultiColumnTree
control on Button click event.
This is my update, I'm sorry for taking a very long time.