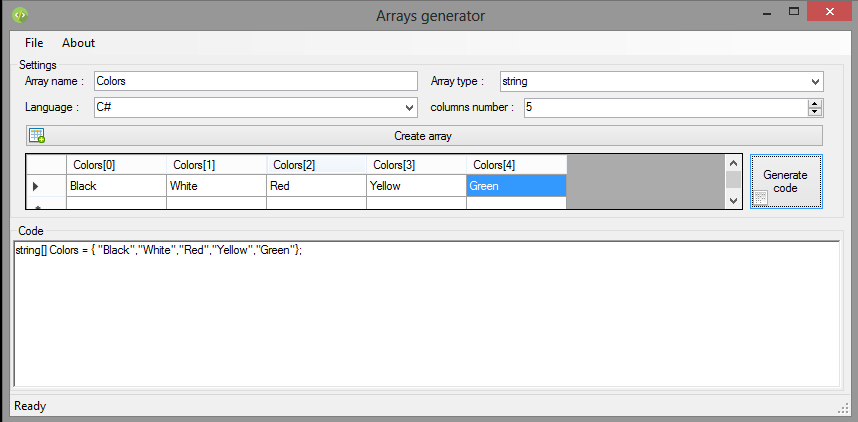
Introduction

This project is a simple application that'll make you able to generate your arrays (long or short ones) in a better and easier way.
Background
While I was creating an application that contains a lot of arrays to highlight some specific words, I felt bored and tired by writing codes and symbols again and again, so I decided to create this project to help me to generate long arrays to save time and strength.
Using the Code
The project uses many loops to:
- Generate a table
- Add items to
ContextMenuStrip
- Generate the array code depending on your settings
So let's explain it part by part.
First, you have to declare some variables that'll contain your settings.
C#
private string arrayName { get; set; }
private string arrayType { get; set; }
private decimal arraySize { get; set; }
private TextBox array = new TextBox();
private string language { get; set; }
VB.NET
Private Property arrayName() As String
Get
Return m_arrayName
End Get
Set(value As String)
m_arrayName = value
End Set
End Property
Private m_arrayName As String
Private Property arrayType() As String
Get
Return m_arrayType
End Get
Set(value As String)
m_arrayType = value
End Set
End Property
Private m_arrayType As String
Private Property arraySize() As Decimal
Get
Return m_arraySize
End Get
Set(value As Decimal)
m_arraySize = value
End Set
End Property
Private m_arraySize As Decimal
Private array As New TextBox()
Private Property language() As String
Get
Return m_language
End Get
Set(value As String)
m_language = value
End Set
End Property
Private m_language As String
Generate a Table
The table you'll generate depends on the settings you entered (example: number of columns):

As I said from the beginning that the project contains many loops, one of them is the one that we'll use to generate the table and the ContextMenuStrip
items:

C#
arrayName = an.Text;
arraySize = number.Value;
arrayType = type.Text;
language = lan.Text;
for (int i = 0; i < arraySize; i++)
{
arrays.Columns.Add(i.ToString(), arrayName + "[" + i + "]");
cm.Items.Add("Go to "+arrayName+"[" + i + "]",
Arrays_generator.Properties.Resources.table_go);
}
VB.NET
arrayName = an.Text
arraySize = number.Value
arrayType = type.Text
language = lan.Text
For i As Integer = 0 To arraySize - 1
arrays.Columns.Add(i.ToString(), arrayName + "[" + i.ToString() + "]")
cm.Items.Add("Go to " + arrayName + "[" + i.ToString() + "]",
ArraysGenerator.My.Resources.table_go)
Next
As you can notice that the code will simply add columns into the datagridview
controller and items (including its image) to the right click popup menu.
As a user, all you have to do is to fill the cells, then generate the code. :)
BUT wait, you are a developer and you want to understand how it works!
Trust me it's too easy, you only have to manipulate strings, and use some if statements. Take a look at a simple code:
C#
if (language == "VB.net")
{
if (arrayType == "string")
{
code.Text = "dim " + arrayName + " as string() = {";
for (int i = 0; i < arrays.Columns.Count; i++)
{
string cellValue = arrays.Rows[0].Cells[i].Value.ToString();
code.AppendText(symbole + cellValue + symbole + ",");
}
code.AppendText("}");
code.Text = code.Text.Replace(",}", "};");
}
}
VB.NET
If language = "VB.net" Then
If arrayType = "string" Then
code.Text = "dim " + arrayName + " as string() = {"
For i As Integer = 0 To arrays.Columns.Count - 1
Dim cellValue As String = arrays.Rows(0).Cells(i).Value.ToString()
code.AppendText((symbole & cellValue) + symbole + ",")
Next
code.AppendText("}")
code.Text = code.Text.Replace(",}", "}")
End If
End If
The result is as follows:

Notes
- You can create your custom generator basing it on this one.
- I did not add syntax highlighting because I wanted it to be as simple as possible.