Introduction
This is a Windows application which processes your code and shows how much time it takes to execute your code.
Using the Code
The application image is shown below:
<a href="http://ptcSuccess.png" target="_self"><img alt="" src="/KB/dotnet/1019082/ptcSuccess.png" style="width: 640px; height: 342px;" /></a>
You should append your code at "//Enter your code here..." section. Once you complete your code editing, click on run button, then you can see how much time your code takes for execution at the bottom(output) tab.
If your code had any errors, then all the error messages will be displayed in the output tab.
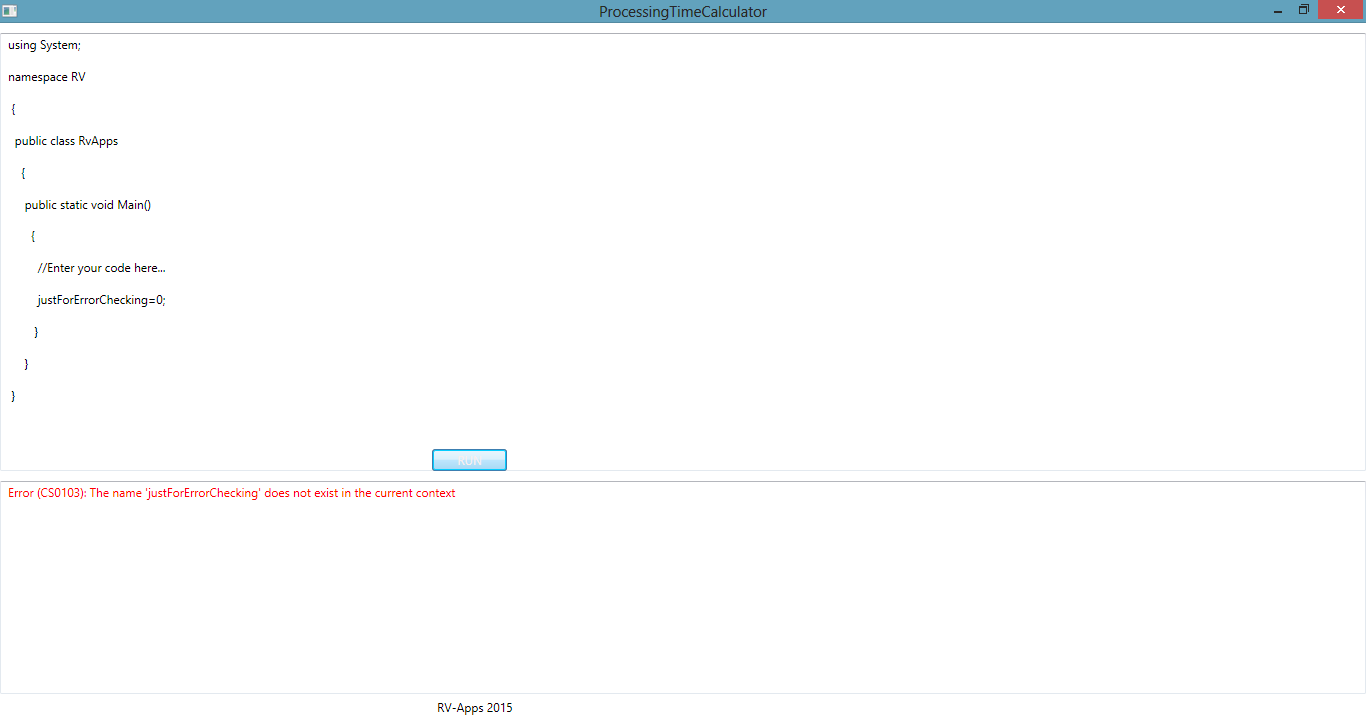
Once we look at the code, we can see how exactly our application works.
using System;
using System.Windows;
using System.Windows.Documents;
using RTenney.Utility.Timekeeping;
using Microsoft.CSharp;
using System.CodeDom.Compiler;
using System.Reflection;
using System.Windows.Controls;
using System.Windows.Media;
namespace ProcessingTimeCalculator
{
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
codeTextAreaRak.AppendText(GetInitialCodeTemplate());
}
public string GetInitialCodeTemplate()
{
string code =
@"using System;
namespace RV
{
public class RvApps
{
public static void Main()
{
//Enter your code here...
}
}
}
";
return code;
}
private void runBtn_Click(object sender, RoutedEventArgs e)
{
codeTextAreaRak.IsReadOnly = true;
string code = new TextRange
(codeTextAreaRak.Document.ContentStart, codeTextAreaRak.Document.ContentEnd).Text;
CSharpCodeProvider provider = new CSharpCodeProvider();
CompilerParameters parameters = new CompilerParameters();
parameters.ReferencedAssemblies.Add("System.Drawing.dll");
parameters.GenerateInMemory = true;
parameters.GenerateExecutable = true;
CompilerResults results = provider.CompileAssemblyFromSource(parameters, code);
if (results.Errors.HasErrors)
{
OutputWindow.Foreground = Brushes.Red;
foreach (CompilerError error in results.Errors)
{
OutputWindow.AppendText
(String.Format("Error ({0}): {1}", error.ErrorNumber, error.ErrorText));
OutputWindow.AppendText("\n");
}
codeTextAreaRak.IsReadOnly = false;
return;
}
OutputWindow.Foreground = Brushes.Green;
Assembly assembly = results.CompiledAssembly;
Type program = assembly.GetType("RV.RvApps");
MethodInfo main = program.GetMethod("Main");
TimeData timer = Timekeeper.StartNew();
main.Invoke(null, null);
Timekeeper.End(timer);
double timeCount = timer.Time;
OutputWindow.AppendText
(DateTime.Now+": Total time of execution " + timeCount+"\n");
codeTextAreaRak.IsReadOnly = false;
}
}
}
CodeTextRak
and OutputWindow
are rich textbox controls. Your code will be appended to CodeTextRak
rich text box and output will be displayed in OutputWindow
rich textbox.
public MainWindow()
{
InitializeComponent();
codeTextAreaRak.AppendText(GetInitialCodeTemplate());
}
Here CodeTextAreaRak
section will be loaded with initial template and all other controls will be initialized.
private void runBtn_Click(object sender, RoutedEventArgs e)
{
codeTextAreaRak.IsReadOnly = true;
string code = new TextRange(codeTextAreaRak.Document.ContentStart,
codeTextAreaRak.Document.ContentEnd).Text;
CSharpCodeProvider provider = new CSharpCodeProvider();
CompilerParameters parameters = new CompilerParameters();
parameters.ReferencedAssemblies.Add("System.Drawing.dll");
parameters.GenerateInMemory = true;
parameters.GenerateExecutable = true;
CompilerResults results = provider.CompileAssemblyFromSource(parameters, code);
if (results.Errors.HasErrors)
{
OutputWindow.Foreground =
Brushes.Red;
foreach (CompilerError error in results.Errors)
{
OutputWindow.AppendText(String.Format("Error ({0}): {1}",
error.ErrorNumber, error.ErrorText));
OutputWindow.AppendText("\n");
}
codeTextAreaRak.IsReadOnly = false;
return;
}
OutputWindow.Foreground =
Brushes.Green;
Assembly assembly = results.CompiledAssembly;
Type program = assembly.GetType("RV.RvApps");
MethodInfo main = program.GetMethod("Main");
TimeData timer = Timekeeper.StartNew();
main.Invoke(null, null);
Timekeeper.End(timer);
double timeCount = timer.Time;
OutputWindow.AppendText(DateTime.Now+": Total time of execution " +
timeCount+"\n");
codeTextAreaRak.IsReadOnly = false;
}
How each line of the code works is clearly explained as a comment next to it.
Points of Interest
Usually, I like to write my code in online compiler sites like codechef, techgig, google codejam. While writing code, I always think of how exactly these sites compile our code and sometimes I think of doing an offline version of it.