Introduction
I have written this tip to show the simple treeview
design in html using CSS 3.0 and JQuery 1.10.2. I heard about the designing the treeview
with infinite parent-child relation is somehow difficult. This is a very simple way to design the treeview
in HTML.
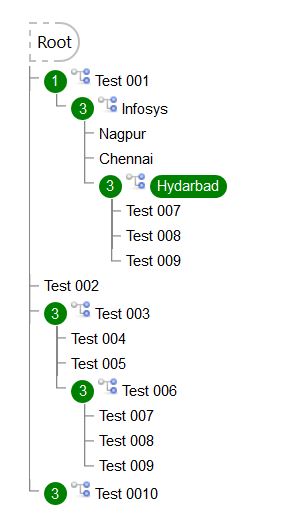
Using the Code
In this tip, I have written a small JavaScript function with two user defined functions.
LoadChildren(ctrl)
NodeSelection(ctrl)
<script type="text/javascript">
function LoadChildren(ctrl) {
if ($(ctrl).next('a').next('ul').is(':visible')) {
$(ctrl).next('a').next('ul').hide('slow');
$(ctrl).attr('src', '/Images/ChildNode.png');
}
else {
$(ctrl).next('a').next('ul').show('slow');
$(ctrl).attr('src', 'Images/ChildNode.png');
}
}
function NodeSelection(ctrl) {
$('ul.tree li').find('a').each(function () { $(this).removeClass(); })
$(ctrl).addClass("selected");
}
$(document).ready(function () {
$('ul.tree li').each(function () {
$(this).children('img').click(function () {
LoadChildren(this);
});
});
$('ul.tree li a').click(function (e) {
$('ul.tree li').find('a').each(function () { $(this).removeClass(); })
$(this).addClass("selected");
});
});
</script>
Function 1 - LoadChildren(ctrl)
is used to load the children nodes dynamically by using Ajax to call the server side function/Action and add the nodes into the corresponding Node. I have written this code for .NET MVC, razor view engine and C# language.
For example:
function LoadChildren(ctrl) {
if ($(ctrl).next('a').next().is('ul') == false) {
var customerID = $(ctrl).attr('id').replace('img_', '');
var nodeStr = '';
var parentNode = $(ctrl).parent('li');
$.ajax({
type: 'post',
url: '@Url.Action("[ActionName]", "[ControllerName]")',
data: { 'CustomerID': parseInt(customerID) },
success: function (childrenObject) {
nodeStr = '<ul>';
$.each(childrenObject.RelationTreeInfo, function (index, data) {
nodeStr += '<li>';
if (data.NoOfChildren != 0) {
nodeStr += '<span>' + data.NoOfChildren + '</span>';
nodeStr += '<img style="margin-left:3px;"
id="img_' + data.CustomerID + '"
src="/Content/Images/ChildNode.png"
onclick="LoadChildren(this)" />';
}
nodeStr += '<a style="margin-left:3px;"
onclick="NodeSelection(this);">' +
data.Firstname + '</a>' + '</li>';
});
nodeStr += '</ul>';
$(parentNode).append(nodeStr);
}
});
}
else {
if ($(ctrl).next('a').next('ul').is(':visible')) {
$(ctrl).next('a').next('ul').hide('slow');
$(ctrl).attr('src', '/Content/Images/ChildNode.png');
}
else {
$(ctrl).next('a').next('ul').show('slow');
$(ctrl).attr('src', '/Content/Images/ChildNode.png');
}
}
}
Points of Interest
Click on icon to collapse and expand the node. Click on node to select the node.