Introduction
As the title suggests, at first I thought it is an easy task. I mean to rename a file/directory from uppercase or any case to lower case form, how hard can that be?
Obviously I am wrong, it is not very difficult but not very trivial either. So here I am ...
Background
The first approach I come up with (I think a lot of us will do):
DirectoryInfo _dirInfo = new DirectoryInfo("Your Path");
_dirInfo.MoveTo( _dirInfo.Fullname.ToLower() );
Voila, I think I am done then, when I run the program, I get an Exception.
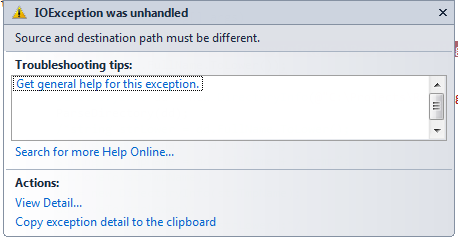
The source and destination path must be different. That make sense, as I only change the directory name to its lower case form.
So to overcome this problem, I revise the
MoveTo
method of the DirectoryInfo
class and come up
with the following:
- If I can't change to lower case, how about I append something to the end?
- Use the newly created path from (1) and re-instantiate
DirectoryInfo
. - Use (2) to call MoveTo again and obtain the result.
static DirectoryInfo RenameDirectoryToLower(DirectoryInfo dirInfo)
{
string strLower = dirInfo.FullName.ToLower();
string strTmpName = dirInfo.FullName + "_temp";
dirInfo.MoveTo(strTmpName);
dirInfo = new DirectoryInfo(strTmpName);
dirInfo.MoveTo(strLower);
return new DirectoryInfo(strLower);
}
A similar approach can be used for renaming a file to its lower case. Since it is a very small program, I just attached the code below:
class Program
{
static void Main(string[] args)
{
var _directoryInfo = new DirectoryInfo(@"C:\testpath");
ParseDirectory(_directoryInfo);
Console.ReadLine();
}
static void ParseDirectory(DirectoryInfo directoryInfo)
{
foreach (var objInfo in directoryInfo.GetDirectories())
{
ParseDirectory(RenameDirectoryToLower(objInfo));
}
foreach (var objFileInfo in directoryInfo.GetFiles())
{
RenameFileToLower(objFileInfo);
}
}
static DirectoryInfo RenameDirectoryToLower(DirectoryInfo dirInfo)
{
string strLower = dirInfo.FullName.ToLower();
string strTmpName = dirInfo.FullName + "_temp";
dirInfo.MoveTo(strTmpName);
dirInfo = new DirectoryInfo(strTmpName);
dirInfo.MoveTo(strLower);
return new DirectoryInfo(strLower);
}
static FileInfo RenameFileToLower(FileInfo fileInfo)
{
string strLower = fileInfo.FullName.ToLower();
string strTmpName = fileInfo.FullName + "_temp";
fileInfo.MoveTo(strTmpName);
fileInfo = new FileInfo(strTmpName);
fileInfo.MoveTo(strLower);
return new FileInfo(strLower);
}
}
That is it! I have managed to change both the directory and file name into lower case form.
Hope you enjoy this small tip. Happy programming.