Introduction
In this article you learn how to create a menu using JavaScript on mouseover and hide this menu on mouse out event.
How to Create a Menu
First of all we need to add a style for a div
which contains the menu and then for
the table's column td
.
<style>
.DivMenu {
position:absolute;
left:-200px;
top:-1000px;
width:180px;
z-index:100;
background-color: darkorange;
border: 1px groove lightgrey;
}
.TDMenu {
color:darkblue;
font-family:verdana;
font-size:70%;
width:100%;
cursor:default;
}
</style>
In the ASPX page you can add any control on which you want to show a menu on mouseover event and hide on
the mouseout event.
Then you create a div
and in this div
use a table, and on both controls
(div
and table's td
) apply the style class.
<div id="JsMenuDiv" class="DivMenu" onmouseout="return hideMenu(this)">
<TD id="MenuSaveAs" RollOver RollOut onclick="goPage('Default4.aspx')" class="TDMenu">
In td, we use Rollover RollOut
. We use it in the JavaScript function; on
the mouseover and mouseout events of this td, we change the color.
function document_onmouseover()
{
var srcElement = event.srcElement;
if (srcElement.tagName == "TD" && typeof(srcElement.RollOver) != "undefined")
{
srcElement.style.color = "white";
srcElement.style.backgroundColor ="darkblue";
}
}
function document_onmouseout()
{
var srcElement = event.srcElement;
if (srcElement.tagName == "TD" && typeof(srcElement.RollOut) != "undefined")
{
srcElement.style.color = "darkblue";
srcElement.style.backgroundColor = "darkorange";
}
}
document.onmouseover = document_onmouseover;
document.onmouseout = document_onmouseout;
Figure 1. When mouse over then backcolor change
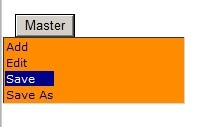
Now we add two functions for showing and hiding menu on mouseover and mouseout events.
function showMenu(menuToShow)
{
var srcElement = event.srcElement;
var xPos = parseInt(srcElement.offsetLeft);
var yPos = parseInt(srcElement.offsetTop);
menuToShow.style.left = xPos + (srcElement.width)
menuToShow.style.top = yPos+40;
}
function hideMenu(menuToHide)
{
if (event.toElement != menuToHide &&
menuToHide.contains(event.toElement) == false)
{
menuToHide.style.left = -200;
menuToHide.style.top = -1000;
}
}
Figure 2. When mouse over on Button then show menu
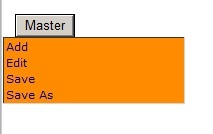