Introduction
The User Datagram Protocol (UDP) is one of the core members of the Internet Protocol Suite, the set of network protocols used for
the Internet. With UDP, computer applications can send messages,
in this case referred to as datagrams, to other hosts
on an Internet Protocol (IP) network without
requiring prior communication to set up special transmission channels or data paths.
This is a Windows application which keeps listening to all unallocated ports and keeps sending to the network through a specific port.
Using the code
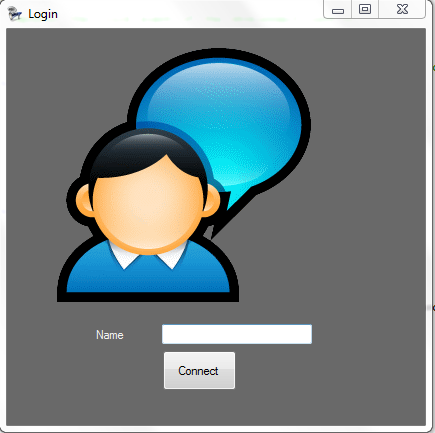
This is the login screen, and the next window is the chat window.

The code used for the login screen:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.Net.Sockets;
using System.Net;
namespace Chat
{
public partial class Login : Form
{
public Login()
{
InitializeComponent();
txtUserName.Focus();
}
private void btnConnect_Click(object sender, EventArgs e)
{
LoginMethod();
}
private void LoginMethod()
{
if (!string.IsNullOrEmpty(txtUserName.Text))
{
UdpClient sendClient = new UdpClient();
Byte[] Text = Encoding.ASCII.GetBytes(txtUserName.Text + " Joined the room...");
IPEndPoint sendEndPoint = new IPEndPoint(IPAddress.Broadcast, 1800);
sendClient.Send(Text, Text.Length, sendEndPoint);
sendClient.Close();
this.Hide();
Message m = new Message();
m.name = txtUserName.Text;
m.Show();
}
}
private void txtUserName_KeyDown(object sender, KeyEventArgs e)
{
if (e.KeyCode == Keys.Enter)
{
LoginMethod();
}
}
private void Login_Load(object sender, EventArgs e)
{
txtUserName.Focus();
}
}
}
The code for the messenger screen:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.Net.Sockets;
using System.Net;
using System.Threading;
namespace Chat
{
public partial class Message : Form
{
UdpClient receiveClient = new UdpClient(1800);
IPEndPoint receiveEndPint = new IPEndPoint(IPAddress.Any, 0);
public string name;
public Message()
{
InitializeComponent();
}
private void Message_Load(object sender, EventArgs e)
{
txtMessageWindow.Text = name + " Joined the room...";
Thread rec = new Thread(ReceiveMessageFn);
rec.Start();
txtTextMessage.Focus();
}
private void ReceiveMessageFn()
{
try
{
while (true)
{
Byte[] receve = receiveClient.Receive(ref receiveEndPint);
string message = Encoding.ASCII.GetString(receve);
if (message == name + " logged out...")
{
break;
}
else
{
if (message.Contains(name + " says >>"))
{
message = message.Replace(name + " says >>", "Me says >>");
}
ShowMessage(message);
}
}
Thread.CurrentThread.Abort();
Application.Exit();
}
catch(ThreadAbortException ex)
{
Application.Exit();
}
}
private void txtTextMessage_KeyDown(object sender, KeyEventArgs e)
{
if (e.KeyCode == Keys.Enter)
{
if (!string.IsNullOrEmpty(txtTextMessage.Text))
{
string data = name + " says >> " + txtTextMessage.Text;
SendMessage(data);
}
}
}
private void SendMessage( string data)
{
UdpClient sendClient = new UdpClient();
Byte[] message = Encoding.ASCII.GetBytes(data);
IPEndPoint endPoint = new IPEndPoint(IPAddress.Broadcast, 1800);
sendClient.Send(message, message.Length, endPoint);
sendClient.Close();
txtTextMessage.Clear();
txtTextMessage.Focus();
}
private void btnSend_Click(object sender, EventArgs e)
{
if (!string.IsNullOrEmpty(txtTextMessage.Text))
{
string data = name + " says >> " + txtTextMessage.Text;
SendMessage(data);
}
}
private void ShowMessage(string message)
{
if (this.InvokeRequired)
this.Invoke(new MethodInvoker(delegate() { ShowMessage(message); }));
else
{
txtMessageWindow.Text = txtMessageWindow.Text + Environment.NewLine + message;
txtMessageWindow.SelectionStart = txtMessageWindow.TextLength;
txtMessageWindow.ScrollToCaret();
txtMessageWindow.Refresh();
}
}
private void Message_FormClosing(object sender, FormClosingEventArgs e)
{
string data = name + " logged out...";
SendMessage(data);
}
private void txtMessageWindow_KeyDown(object sender, KeyEventArgs e)
{
e.SuppressKeyPress = true;
}
}
}