Introduction
FREE for PERSONAL and COMMERCIAL use
The SolidWidgets
UI framework includes a powerful GRID control that can be bound to a database table, and to cached memory. The memory mode of the grid allows for unlimited
number of rows to be added to the grid from any external source, and the database mode fetches its data directly from the database, and also allows for unlimited number of rows to be displayed
and manipulated. The grid can be configured for up to 255 columns, and each column can be configured to be readonly, editable, editing style, which includes simple text edit, combobox, checkbox,
button, image, spinner, masked, numeric, etc. The grid can also be configured to display all the columns by scaling them according to the size that you set for each column, or it can display
whatever number of columns that can be fit on the current screen, you would then scroll left and right to view the rest of the columns. These are just few of the features
that are supported in this grid.
Using the SolidWidgets GRID class
In order to add a grid to a window in your application, first declare an instance of swTable
, configure the grid through this instance,
and then add the instance to the window. Following is an example of how a grid is addd and manipulated:
Let's create a dialog class that will display our grid. The dialog class will be made up of the header (GridDemo.h), and the implementation file
(GridDemo.cpp). Here are the contents of the 2 files:
[GridDemo.h]
#pragma once
#include <swtable.h>
#include <swDialog.H>
#include <swbutton.h>
#include <swVector.h>
class GridDemo: public swDialog
{
swTable m_grid;
swButton m_okBtn,m_cancelBtn;
public:
void windowOpening();
void windowOpened();
BOOL windowClosing();
void windowClosed();
void actionPerformed(long sourceId,long eventId,const swString& eventName);
};
Now here is the implementation file of our dialog class:
[GridDemo.cpp]
#include "GridDemo.h"
void GridDemo::windowOpening()
{
setSize(800,600);
swPanel *contentPane = getContentPane();
if(contentPane!=NULL)
{
contentPane->setMargins(10,10,10,10);
contentPane->addRow();
contentPane->addRow(10);
contentPane->addRow(25);
contentPane->addColumn();
contentPane->addColumn(100); contentPane->addColumn(100);
contentPane->addChild(&m_grid,0,0,3,1);
contentPane->addChild(&m_okBtn,1,2,1,1);
contentPane->addChild(&m_cancelBtn,2,2,1,1);
}
m_grid.setTitle(L"Grid Demo");
m_grid.setColumnWidthByRatio(TRUE);
m_grid.setAllowSearch(TRUE);
m_grid.addColumn(L"Name",L"Name",20,2,TRUE,TEXTEDIT);
m_grid.addColumn(L"Gender",L"Gender",20,1,TRUE,COMBOEDIT);
{
swVector<KeyValue> genderOptions;
genderOptions.push_back(KeyValue(L"M",L"Male"));
genderOptions.push_back(KeyValue(L"F",L"Female"));
m_grid.setComboColumnOptions(L"Gender",genderOptions);
}
m_grid.addColumn(L"IsSenior",L"Is Senior",1,1,TRUE,CHECKEDIT);
m_grid.addColumn(L"Age",L"Age",5,1,TRUE,SPINEDIT);
{
swVector<KeyValue> ageOptions;
for(long i=0;i<125;i++)
{
KeyValue newVal(L"",L"");
newVal.key.format(L"%d",i);
newVal.value.format(L"%d",i);
ageOptions.push_back(newVal);
}
m_grid.setSpinColumnOptions(L"Age",ageOptions);
}
m_grid.addColumn(L"BirthDate",L"DOB",12,1,TRUE,DATEEDIT);
m_grid.addColumn(L"Income",L"Income",20,1,TRUE,NUMERICEDIT);
m_grid.addColumn(L"Telephone",L"Telephone",20,1,TRUE,MASKEDIT,L"(###) ###-####");
m_grid.addColumn(L"Options",L"Other Options",20,1,TRUE,BUTTONEDIT);
m_grid.bindToCache();
if(m_grid.addRow())
{
m_grid.setColumnValue(L"Name",L"John Doe");
}
if(m_grid.addRow())
{
m_grid.setColumnValue(L"Name",L"Jane Doe");
}
m_okBtn.setActionListener(this);
m_okBtn.setText(L"OK");
m_cancelBtn.setActionListener(this);
m_cancelBtn.setText(L"Cancel");
}
void GridDemo::windowOpened()
{
}
BOOL GridDemo::windowClosing()
{
return TRUE;
}
void GridDemo::windowClosed()
{
}
void GridDemo::actionPerformed(long sourceId,long eventId,const swString& eventName)
{
if(sourceId == m_okBtn.getID())
{
dispose(swOK);
}
else if(sourceId == m_cancelBtn.getID())
{
dispose(swCANCEL);
}
}
Final Outcome:
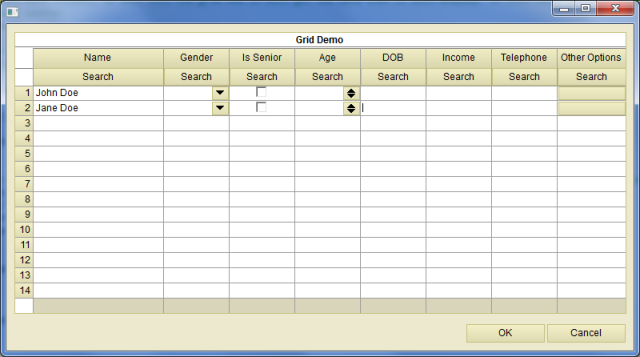
Points of Interest
As easy and simplistic as this code looks, you will start realizing the power of this framework as you dig deeper into the various components.
You can find more help on http://www.solidwidgets.com. The site includes a bunch of examples on how to use each component.
History
I hope this tutorial helps someone who needs to implement such functionality. Best of luck!