Introduction
In this article I will tell you how to send email using a WCF Service. We can send email directly from the client; however, in some cases you can't send external emails directly. In that case we need a WCF service for sending an email from the client. In this article, I will first explain how to create a WCF service then try to implement email functionality using the WCF Service. In this article I am using GMail to send email. So the user must have a GMail account for sending emails.
Background
I have already written so many articles on WCF:
Using the code
1. Create WCF Service Class Library
Create a new Project WCF Class Library Project and Name it EmailServices
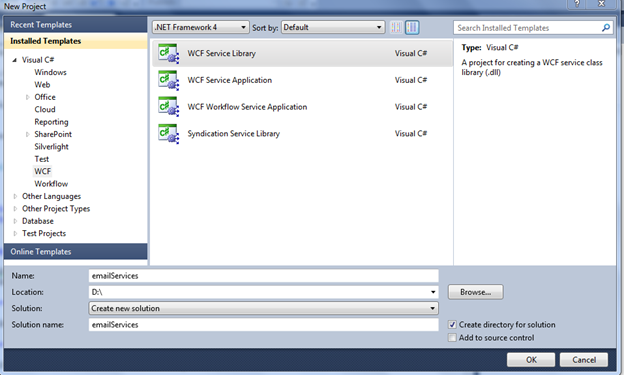
Step 1: Create service contract as IEmailService
and add OperationContract SendEmail
for sending email. This method uses GMail user address, password, and email to, cc, and subject as parameters:
[ServiceContract]
public interface IEmailService {
[OperationContract]
string SendEmail(string gmailUserAddress, string gmailUserPassword,
string[] emailTo,string[] ccTo, string subject, string body, bool isBodyHtml);
}
Step 2: Add the EmailService
class and implement IEmailService
.
public class EmailService : IEmailService
{
private static string SMTPSERVER = "smtp.gmail.com";
private static int PORTNO = 587;
public string SendEmail(string gmailUserName, string gmailUserPassword,
string[] emailToAddress, string[] ccemailTo, string subject, string body, bool isBodyHtml)
{
if (gmailUserName == null || gmailUserName.Trim().Length == 0)
{
return "User Name Empty";
}
if (gmailUserPassword == null || gmailUserPassword.Trim().Length == 0)
{
return "Email Password Empty";
}
if (emailToAddress == null || emailToAddress.Length == 0)
{
return "Email To Address Empty";
}
List<string> tempFiles = new List<string>();
SmtpClient smtpClient = new SmtpClient(SMTPSERVER, PORTNO);
smtpClient.EnableSsl = true;
smtpClient.DeliveryMethod = SmtpDeliveryMethod.Network;
smtpClient.UseDefaultCredentials = false;
smtpClient.Credentials = new NetworkCredential(gmailUserName, gmailUserPassword);
using (MailMessage message = new MailMessage())
{
message.From = new MailAddress(gmailUserName);
message.Subject = subject == null ? "" : subject;
message.Body = body == null ? "" : body;
message.IsBodyHtml = isBodyHtml;
foreach (string email in emailToAddress)
{
message.To.Add(email);
}
if (ccemailTo != null && ccemailTo.Length > 0)
{
foreach (string emailCc in ccemailTo)
{
message.CC.Add(emailCc);
}
}
try
{
smtpClient.Send(message);
return "Email Send SuccessFully";
}
catch
{
return "Email Send failed";
}
}
}
}
Step 3: Build your Class Library.
2. Add WCF Service Application Project

Step 1: Add reference of Class Library Project to that WCFServiceHost project.
Step 2: Delete IService1.Cs and the code-behind file.
Step 3: Right click on that SaleService.svc and select view Markup:

Step 4: Change the Service Name from that markup:
<%@ ServiceHost Language="C#" Debug="true"
Service="EmailServices.EmailService" CodeBehind="EmailService.svc.cs" %>
Step 5: And build the solution and WCFServiceHost
set as startup project and EmailService.svc set as startup page and run the service.

3. Testing Email Service
- Use WCFSTORM for testing WCF Service . You can download WCFSTORM from http://www.wcfstorm.com/wcf/home.aspx.
- Open WCFStrom and add the following endpoint http://localhost:53818/EmailService.svc?wsdl.

By using this, you have successfully send email using WCF Service.

Please see the attached source code.
Happy programming!!
If you have any queries, Reply Me