Introduction
To display grid with pagination and sorting functionality in an easy way, I have created a plugin named jGrid
.
In this tutorial, I am trying to explain this new plugin. This is a small JavaScript library which enables us to create grid with pagination and sorting functionality. This plugin facilitates retrieving page wise data from server.
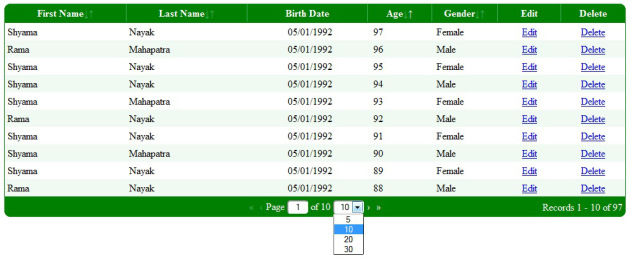
Advantages
- It is very easy to implement.
- It works with any lower or higher versions of jQuery plugin.
- It works with any mainstream browser.
- Grid rendering is fast because it uses only
<div>
HTML tag to display grid. - No image is needed to implement.
Implementation
Before going to implement, we need to download jGrid plugin and CSS files.
Then add these files in your project folders.
This plugin depends on jQuery plugin. So first, you need to add reference of jQuery plugin.
<script src="http://code.jquery.com/jquery-1.10.1.min.js" type="text/javascript"></script>
Then add reference of jGrid plugin and CSS file.
<script type="text/javascript" src="Scripts/jgrid-1.0.js"></script>
<link href="Styles/jgrid.css" rel="stylesheet" />
Put <div>
tag in your page.
<div id="divGrid">
</div>
To make this div
as grid with sorting and pagination functionality, you will write in JavaScript file as:
$(function () {
$("#divGrid").jGrid({
url: '/Service1.svc/GetPersons',
columns: [
{ title: "First Name", index: "FirstName", width: '20' },
{ title: "Last Name", index: "LastName", width: '20' },
{ title: "Birth Date", index: "DateOfBirth", width: '20', align: 'center', sortable: false },
{ title: "Age", index: "Age", width: '10' },
{ title: "Gender", index: "Gender", width: '10' },
{ title: "Edit", index: "Edit", width: '10', align: 'center', sortable: false, formatter: editLink },
{ title: "Delete", index: "Delete", width: '10', align: 'center', sortable: false, formatter: deleteLink }
],
width: '1000',
pageSizes: [5, 10, 20, 30],
rows: 10,
sortName: 'Age',
sortOrder: 'desc'
});
});
function editLink(rowValue) {
return '<a href="#">Edit</a>';
}
function deleteLink(rowValue) {
return '<a href="#">Delete</a>';
}
HTML
The HTML file will look like this:
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
<script src="http://code.jquery.com/jquery-1.10.1.min.js" type="text/javascript"></script>
<script type="text/javascript" src="Scripts/jgrid-1.0.js"></script>
<link href="Styles/jgrid.css" rel="stylesheet" />
<script type="text/javascript">
$(function () {
$("#divGrid").jGrid({
url: '/Service1.svc/GetPersons',
columns: [
{ title: "First Name", index: "FirstName", width: '20' },
{ title: "Last Name", index: "LastName", width: '20' },
{ title: "Birth Date", index: "DateOfBirth", width: '20', align: 'center', sortable: false },
{ title: "Age", index: "Age", width: '10' },
{ title: "Gender", index: "Gender", width: '10' },
{ title: "Edit", index: "Edit", width: '10', align: 'center', sortable: false, formatter: editLink },
{ title: "Delete", index: "Delete", width: '10', align: 'center', sortable: false, formatter: deleteLink }
],
width: '1000',
pageSizes: [5, 10, 20, 30],
rows: 10,
sortName: 'Age',
sortOrder: 'desc'
});
});
function editLink(rowValue) {
return '<a href="#">Edit</a>';
}
function deleteLink(rowValue) {
return '<a href="#">Delete</a>';
}
</script>
</head>
<body>
<form id="form1" runat="server">
<div id="divGrid">
</div>
</form>
</body>
</html>
The server side code can be webmethod, WCF methods or web API methods.
You should pass four parameters (sidx
, sord
, page
, rows
) to the server side method.
public CustomClass GetPersons(string sidx, string sord, int page, int rows)
{
return new CustomClass
{
totalPages = totalPages,
rows = personList,
totalRecords = totalRecords
};
}
Please note that the return result format should be:
return new CustomClass
{
totalPages = totalPages,
rows = personList,
totalRecords = totalRecords
};
History
In this tutorial, I have explained the advantages and features of the plugin jGrid. This plugin now includes only sorting and paging features. I will include more features in the next version of this plugin later.
You can download the demo project from the link at the top of the tip.