Introduction
This tip shows how we can add Editable Template inside a Kendo Grid Column. Technology included ASP.NET MVC, jQuery and Kendo Controls.
Background
Recently, we faced this challenge in our business application, where we needed Grid Column Editable, but what type of value user can choose depends on XML configuration - more specifically depends on User Name and values in other Grid Column.
Here is how my Grid looks like:
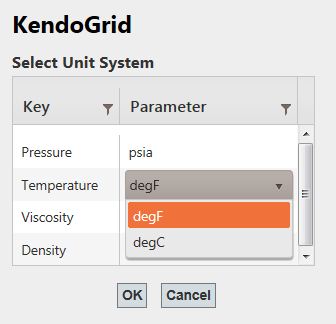
Using the Code
Binding Kendo Grid with Model data in MVC is straightforward (Refer to the attached file for more).
Jumping to the next step, we made one column editable in Grid.
My Model Class consumes XML data.
="1.0"="utf-8"
<Custom>
<PropertyType Name="Pressure"
Value="psia,bara" SelectedValue="psia"></PropertyType>
<PropertyType Name="Temperature"
Value="degF,degC" SelectedValue="degF"></PropertyType>
<PropertyType Name="Viscosity"
Value="cP" SelectedValue="cP"></PropertyType>
<PropertyType Name="Density"
Value="lb/ft3,kg/m3" SelectedValue="lb/ft3"></PropertyType>
</Custom>
We added one Read-Only property in Model class - AvailableValues
. This will just return List of Values to be displayed in DropDown
and mapped with Value
attribute in XML above.
public List<string> AvailableValues
{
get
{
if (!string.IsNullOrEmpty(Value))
{
return Value.Split(',').ToList();
}
return null;
}
}
Here is our MVC Grid. We bound "Parameter
" column with AvailableValues
and ClientTemplate
should display SelectedValue
property.
@(Html.Kendo().Grid(Model.PropertyType).Name("EditableGrid")
.Columns(columns =>
{
columns.Bound(p => p.Name).Title("Key").Width(60);
columns.Bound(p => p.AvailableValues)
.ClientTemplate("#=SelectedValue#")
.Title("Parameter")
.EditorTemplateName("AvailableValues").Width(100);
})
.Editable(editable => editable.Mode(GridEditMode.InCell))
.Sortable()
.Scrollable(scr => scr.Height(140))
.Filterable()
.DataSource(dataSource => dataSource.Ajax()
.PageSize(20)
.ServerOperation(false)
.Model(model =>
{
model.Id(p => p.Name);
model.Field(p => p.Name).Editable(false);
model.Field(p => p.AvailableValues).Editable(true);
})
)
)
We used EditorTemplateName
as "AvailableValues
". This we declared inside Views/Shaed/EditorTemplated in MVC structure.
@using Kendo.Mvc.UI
@(Html.Kendo().DropDownList()
.Name("Unit")
.DataSource(d => d.Read(r => r.Action
("GetTypes", "Home").Data("getParentID()")))
.DataValueField("Value")
.DataTextField("Text")
.Events(e =>
{
e.Select("onSelect");
})
)
Problem with Editable dropdown is it cannot read client side data available inside "AvailableValues
". So using MVC, we are calling Controller method "GetTypes
". It will receive "AvailableValues
" list and return JSON to bind with DropDown
. This call will happen only when user clicks on Editable column, aka try to change values.
getParentID()
is a JavaScript function used to pull data of available values from Kendo Grid Column.
A small trick I used to apply the Selected Value in Drop Down to be applied on Grid Column on DropDown selection change.
var dataItem;
function getParentID() {
var row = $(event.srcElement).closest("tr");
var grid = $(event.srcElement).closest("[data-role=grid]").data("kendoGrid");
dataItem = grid.dataItem(row);
var arr = [];
$(dataItem.AvailableValues).each(function () {
arr.push(this);
});
return { values: arr };
}
function onSelect(e) {
var selectedValue = this.dataItem(e.item.index()).Value;
if (dataItem != null) {
dataItem.SelectedValue = selectedValue;
}
}
Please refer to the attached for entire code.
Please feel free to raise any problem/concern/query.
Points of Interest
Kendo controls are relatively new to me and I am looking forward to exploring more tricks.
History
- 6th December, 2014: Initial version