Introduction
Anoncrypt by HTCoders is a service for securing text messages in an easy way. It could encrypt your message using strong encryption algorithms, so it will be more secure to send or store, than in plain text. Anyone who will get access to the encrypted message will be unable to read the original message without knowing your password.
Most of our communication channels can be easily accessed by third-parties, not only government and your internet provider, but even by your friends and family (if we're talking about social networks or your personal computer).
Using Anoncrypt, you could encrypt sensitive information with secure AES cipher, so nobody will get access to it without knowing the original password.
Background
In this modern world, unauthorized persons are trying to mine data/view the data illegally.
This project will allow only authorized person to view the data who knows the secret key. So, unauthorized persons will be restricted to some extent.
Module 1: Symmetric Key Algorithm
Symmetric key algorithms are algorithms for cryptographic keys for both encryption of plaintext and decryption of cipher text. The keys may be identical or there may be a simple transformation to go between the two keys. The keys, in practice, represent a shared secret between two or more parties that can be used to maintain a private information link. Symmetric key algorithms are algorithms for cryptography that use the same
Module 2: Self-Destructive Messaging Service
This module is mainly constructed with the idea of providing data security. In this module, the user is provided a textbox to input his data to encipher it and a password. After entering the data in the input field and his password, the user can encrypt it. As the user enters the encrypt button, the user will be provided a link without which the recipient will not be able to decrypt it. This link can be used only once and after using it, the message will be automatically destroyed, that is why it is also called as “Self Destructible Messaging System”.
Modules of Anoncrypt
Design: High level Architecture
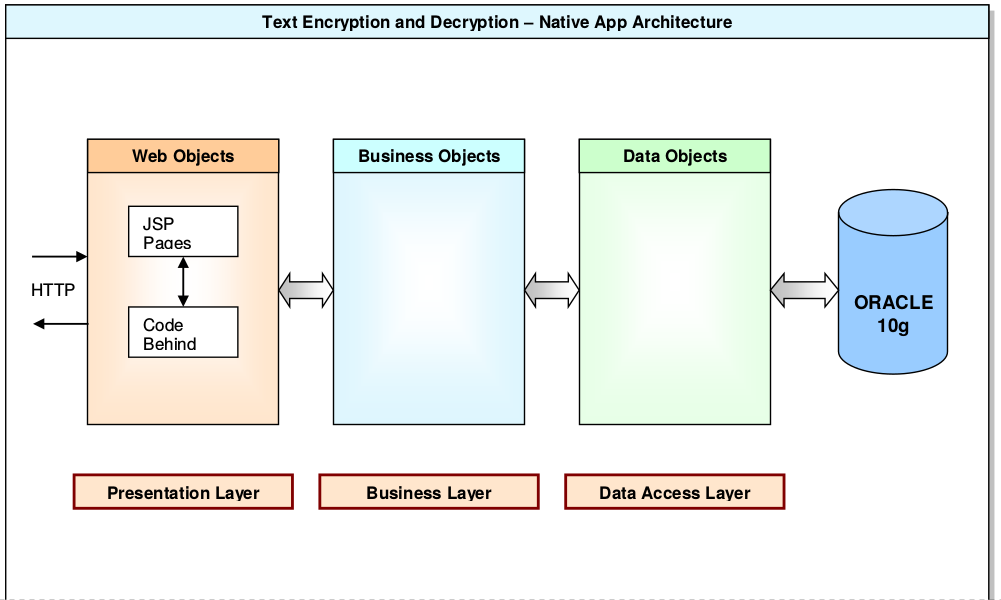
Use Case

Class Digram


Screenshots
1. Screenshot of Home Page

2. Password-based Encryption

3. Access Denied

4. Self-Destructive Message Service

Sample Source Code
package com.anoncrypt.services;
import java.security.Key;
import javax.crypto.Cipher;
import javax.crypto.spec.SecretKeySpec;
import sun.misc.BASE64Decoder;
import sun.misc.BASE64Encoder;
public class SymAES
{
private static final String ALGORITHM = "AES";
private static byte[] keyValue= new byte[] { 'T', 'h', 'i',
's', 'I', 's', 'A', 'S', 'e',
'c', 'r', 'e', 't', 'K', 'e', 'y' };
public String encode(String valueToEnc) throws Exception {
System.out.println("The Key byte value"+keyValue );
Key key = generateKey();
Cipher c = Cipher.getInstance(ALGORITHM);
c.init(Cipher.ENCRYPT_MODE, key);
byte[] encValue = c.doFinal(valueToEnc.getBytes());
String encryptedValue = new BASE64Encoder().encode(encValue);
return encryptedValue;
}
public String decode(String encryptedValue) throws Exception {
try{
Key key = generateKey();
Cipher c = Cipher.getInstance(ALGORITHM);
c.init(Cipher.DECRYPT_MODE, key);
byte[] decordedValue = new BASE64Decoder().decodeBuffer(encryptedValue);
byte[] decValue = c.doFinal(decordedValue);
String decryptedValue = new String(decValue);
return decryptedValue;
}
catch(Exception e)
{
String decryptedValue = new String("no");
return decryptedValue;
}
}
private static Key generateKey() throws Exception {
System.out.println("The Key byte value inside genkey"+keyValue );
Key key = new SecretKeySpec(keyValue, ALGORITHM);
return key;
}
public void start(String passcode)throws Exception
{
int temp=passcode.length();
for(int i=temp;i<32;i++)
{
passcode=passcode+'a';
}
System.out.println("Updated byte "+passcode);
keyValue = passcode.getBytes();
System.out.println("passcode"+passcode);
System.out.println("The Key byte value inside start"+keyValue );
}
}
Presentation
Here is the link for the Presentation related to project:
History
- 6th May, 2015: Initial version
This project was developed when I was a student at University of Allahabad, India.
Contact
For further queries, please visit http://htcoders.blogspot.com/.