Introduction
This article will present how to search and display Youtube videos by channel, keywords and other search terms with PHP and Youtube Data API v3.
Using the code
You can see related documentation and compose own request here: https://developers.google.com/youtube/v3/docs/search/list
The composed request return with json data, this includes the videos information.
Read the json(the part property may be only snippet at current example):
Related documentation: http://php.net/manual/en/function.json-decode.php
$channelId = 'UCVHFbqXqoYvEWM1Ddxl0QDg';
$maxResults = 8;
$API_key = '{YOUR_API_KEY}';
$video_list = json_decode(file_get_contents('https://www.googleapis.com/youtube/v3/search?order=date&part=snippet&channelId='.$channelId.'&maxResults='.$maxResults.'&key='.$API_key.''));
The success response body contains data with following structure(this is one example file):
https://raw.githubusercontent.com/magyarandras/YoutubeVideoList/master/list/example.json
Display videos and playlists:
foreach($video_list->items as $item)
{
if(isset($item->id->videoId)){
echo '<li id="'. $item->id->videoId .'" class="col-lg-3 col-sm-6 col-xs-6 youtube-video">
<a href="#'. $item->id->videoId .'" title="'. $item->snippet->title .'">
<img src="'. $item->snippet->thumbnails->medium->url .'" alt="'. $item->snippet->title .'" class="img-responsive" height="130px" />
<h2>'. $item->snippet->title .'</h2>
<span class="glyphicon glyphicon-play-circle"></span>
</a>
</li>
';
}
else if(isset($item->id->playlistId))
{
echo '<li id="'. $item->id->playlistId .'" class="col-lg-3 col-sm-6 col-xs-6 youtube-playlist">
<a href="#'. $item->id->playlistId .'" title="'. $item->snippet->title .'">
<img src="'. $item->snippet->thumbnails->medium->url .'" alt="'. $item->snippet->title .'" class="img-responsive" height="130px" />
<h2>'. $item->snippet->title .'</h2>
<span class="glyphicon glyphicon-play-circle"></span>
</a>
</li>
';
}
}
I use Bootstrap UI framework(http://getbootstrap.com) for responsive display, and video list template(http://bootsnipp.com/snippets/featured/video-list-thumbnails).
Show Youtube players with jQuery:
$(".youtube-video").click(function(e){
$(this).children('a').html('<div class="vid"><iframe width="420" height="315" src="https://www.youtube.com/embed/'+ $(this).attr('id') +'?autoplay=1" frameborder="0" allowfullscreen></iframe></div>');
return false;
e.preventDefault();
});
$(".youtube-playlist").click(function(e){
$(this).children('a').html('<div class="vid"><iframe width="420" height="315" src="https://www.youtube.com/embed/videoseries?list='+ $(this).attr('id') +'&autoplay=1" frameborder="0" allowfullscreen></iframe></div>');
return false;
e.preventDefault();
});
The result:
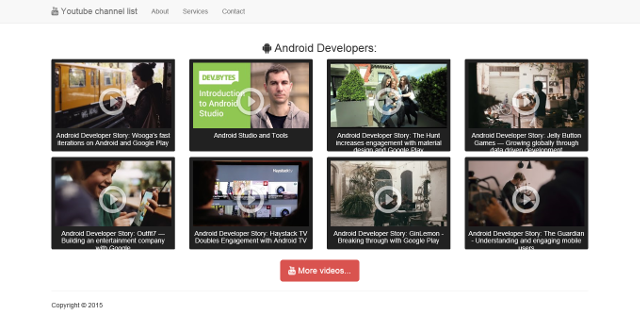
History
Many examples can be found for this purpose, but almost all use the old APIs, therefore i hope useful this solution with the Data API v3.