Introduction
The built-in DataGrid
control in ASP.NET is great for displaying tabular data. This article shows how we can extend this control and modify the pager to display some additional information about the data, such as number of records and number of pages.
Background
The pager in a DataGrid
is helpful in allowing users to page through long lists of data. However, with the page numbers aligned to the right, we have a big empty space to the left which can be used more efficiently (See image 1). This article shows how we can insert some additional information about the data into this space - i.e. a text like: Displaying 82 records. Currently on page 2 of 10. We can also apply some optional formatting, removing the page numbers if there is only one page.
Image 1. The default DataGrid
control.
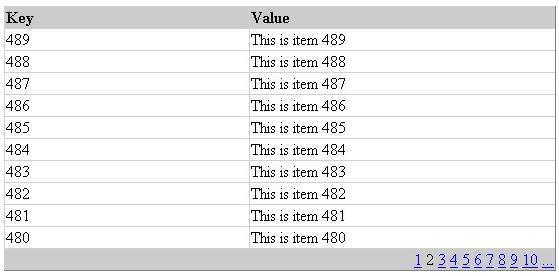
Creating the extended datagrid control
To get started, we create a new class inheriting from the DataGrid
. We name this new class DataGridWithImprovedPager
.
class DataGridWithImprovedPager: DataGrid
{
private bool m_DisplayPageNumbers = true;
public DataGridWithImprovedPager()
{
}
}
We override the OnItemCreated
method, and insert a blank label (and cell) into the pager. We will retrieve this label before the control is rendered and populate it with information about the data being displayed.
protected override void OnItemCreated(DataGridItemEventArgs e)
{
switch (e.Item.ItemType)
{
case (ListItemType.Pager):
m_DisplayPageNumbers = (this.PageCount > 1);
TableCell cell = e.Item.Controls[0] as TableCell;
cell.Visible = m_DisplayPageNumbers;
if (cell.FindControl("lblNumRecords") == null)
{
int count = (m_DisplayPageNumbers && this.Columns.Count > 1) ?
this.Columns.Count - 1 : this.Columns.Count;
TableCell pager = (TableCell) e.Item.Controls[0];
TableCell newcell = new TableCell();
newcell.ColumnSpan = count;
newcell.HorizontalAlign = HorizontalAlign.Left;
newcell.Style["border-color"] = pager.Style["border-color"];
Label lblNumRecords = new Label();
lblNumRecords.ID = "lblNumRecords";
if (this.Columns.Count > 1)
{
newcell.Controls.Add(lblNumRecords);
e.Item.Controls.AddAt(0, newcell);
pager.ColumnSpan = pager.ColumnSpan - count;
}
else
{
cell.Controls.AddAt(0, lblNumRecords);
}
}
break;
}
base.OnItemCreated (e);
}
The final step is to override the OnPreRender
method, and retrieve the label we added previously. We then populate it with the number of items we have in the list, and if we have more than one page, then information about the current page and the total page count is also included.
protected override void OnPreRender(System.EventArgs e)
{
Control c1 = this.Controls[0];
Control c2 = c1.Controls[c1.Controls.Count - 1];
Label lblNumRecords = c2.FindControl("lblNumRecords") as Label;
lblNumRecords.Text = string.Format("{0} records found.", this.VirtualItemCount);
if (m_DisplayPageNumbers)
{
lblNumRecords.Text += string.Format(" Currently on page {0} of {1}.",
CurrentPageIndex+1,
PageCount);
}
base.OnPreRender (e);
}
Image 2. Our improved DataGrid
control.

Points of Interest
I had some trouble retrieving the total number of items in the list from within the DataGrid
, so the VirtualItemCount
property must be set manually from within the code when binding data.
History
This is my first article, and any suggestions or comments would be appreciated! :-)