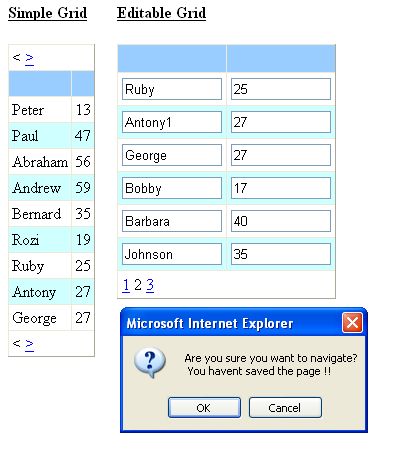
Introduction
Did you know that you can call any JavaScript function or stop the user from moving to the next page when he clicks on the paging links in an ASP.NET DataGrid
? For your information, by default, the paging parts in an ASP.NET DataGrid
are generated by the .NET framework and you don't have any control over it.
Problem
I was once having an editable grid and wanted to make sure that if a user changes the data, he is alerted about it if he tries to go out of the page without saving the change. I was able to put a boundary validation everywhere on the web page, but I wasn't getting how to put it on the DataGrid
paging facility (it is not even controlled through the footer or header item-bound).
How I solved it
I realized that it could only be managed if I use a custom data grid control and manage the paging myself on its OnRender
event, and this is what I have done to mitigate this problem.
Benefits
You can not only alert a user that he is navigating out of the page but also confirm for save changes if he hasn't saved the information while navigating to a different page.
Technical part
How I did it
I created my own custom DataGrid
control and added an attribute BeforePagingCallJSFunction
. You can pass any JavaScript function in this attribute or you can stop the navigation process to different page, by returning false
.
public class DataGridCustom : System.Web.UI.WebControls.DataGrid
{
..
public string BeforePagingCallJSFunction
..
protected override void Render(HtmlTextWriter output)
{
if ( AllowPaging == true && BeforePagingCallJSFunction != "" )
{
System.Text.StringBuilder sb =
new System.Text.StringBuilder();
HtmlTextWriter writer =
new HtmlTextWriter(new System.IO.StringWriter(sb));
base.Render(writer);
string datgridString = sb.ToString();
if ( this.PagerStyle.Position == PagerPosition.Top ||
this.PagerStyle.Position == PagerPosition.TopAndBottom )
{
int firstRowPos = datgridString.IndexOf(@"</tr>");
string firstpart = datgridString.Substring(0,firstRowPos);
string secondpart = datgridString.Substring(firstRowPos);
firstpart = firstpart.Replace("href=\"javascript:__doPostBack(",
( "style='cursor:hand;text-decoration:underline;" +
"color:blue' href1='javascript:void(0)'" +
" onclick=\"javascript:" +
beforePagingCallJSFunction + ";__doPostBack("));
datgridString = firstpart + secondpart;
}
if ( this.PagerStyle.Position == PagerPosition.Bottom ||
this.PagerStyle.Position== PagerPosition.TopAndBottom)
{
int lastRowPos = datgridString.LastIndexOf("<tr");
string firstpart = datgridString.Substring(0,lastRowPos);
string secondpart = datgridString.Substring(lastRowPos);
secondpart = secondpart.Replace("href=\"javascript:__doPostBack(",
( "style='cursor:hand;text-decoration:underline;" +
"color:blue' href1='javascript:void(0)' " +
"onclick=\"javascript:" +
beforePagingCallJSFunction + ";__doPostBack("));
datgridString = firstpart + secondpart;
}
output.Write(datgridString.ToString());
}
else
{
base.Render(output);
}
}
}
How to use it
In ASPX, get the proper references, enable paging (AllowingPaging=true
) and do it as follows:
-
<Tittle:DataGridCustom BeforePagingCallJSFunction =
"alert('You are navigating out of this page')"
..
>
<columns>
</columns>
</Tittle:DataGridCustom>
-
<!--
-
<!--
Some Technical FAQs
- Why did I replace "
href
" with "onclick
"?
It is because stopping the execution of the next JavaScript function is only possible through "onclick
".
- Is there any disadvantage when I use it?
Yes, since I replaced "href
" with "onclick
", you can not focus on paging hyperlink through Tab.
- Will this work in case I have paging on top, bottom or both?
Yes.
Future
- You can even check which page number the user has clicked, by reading the
innerText
of the hyperlink and passing this to the JavaScript function called.
- You can replace the hard-coded
__doPostBack
with Page.GetPostBackEventReference
in case it is changed in future.
- You can format or color the paging text the way you want.
Conclusion
Not necessarily every one will like it, but it is for developers who have ever tried implementing boundary handling in their web applications, because this is a place they never had control earlier while validating if the page content was saved or not, here they can now.
Please free to post your comments and feedback.