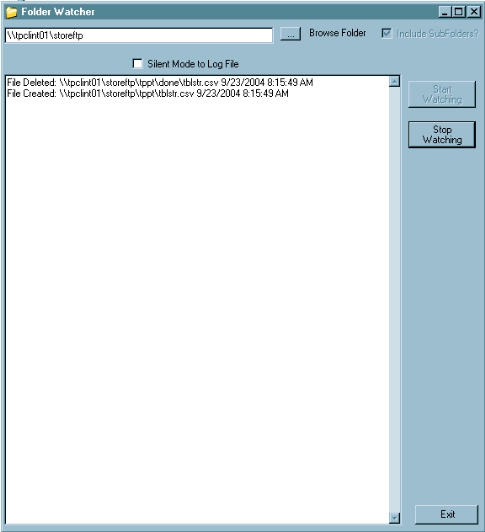
Introduction
The Microsoft FileSystemWatcher
class is pretty cool, I use it to see when new files are ready to be processed, and it works pretty good. However, the FileSystemWatcher
does have its limitations. The one limitation that has caused me to write this code, and the article, is the FileSystemWatcher
has no ability to tell the client that a folder does not exist anymore.
In one of my programs, I am watching a network folder. The program starts, and stays running 24x7x365. It is supposed to detect new files, and then other processes take the file and import or export it based on what type of file it is. The process of detecting files is mission critical, and needs to keep running and be able to recover after an error.
We had network problems last week, and the FileSystemWatcher
has no current way of notifying the user or application that the folder/path we are watching is no longer available. And after the network path is restored, the FileSystemWatcher
does not continue to watch the folders, and throws no errors or indication that the network path is unavailable, or that it has been restored.
Problem
When a network outage occurs, the FileSystemWatcher
has no event that it fires to tell the application that the folder/path cannot be accessed. And, it has no way to recover once the network has been restored.
Solution
What I did was inherit the base FileSystemWatcher
class, and add some custom events, a property, and a timer. By inheriting the base class, all the original functionality is retained. By adding events, a timer, and a property, we can use the timer_elapsed
event to look and see if the network path exists. The property is an interval for the timer, so the user can tell the new class how often it should look to see if the network is still available. If the user does not specify an interval, the default 100 millisecond interval is used.
I added two new public events: NetworkPathAvailable
and NetworkPathUnavailable
. In code, the user will look in these events to determine if the network path is available, and the code can take the appropriate measures to continue working, or die gracefully.
The AdvancedFileSystemWatcher
class can be used in .NET 1.1 or 2.0; the code I have provided is for VS2005 and .NET 2.0, but you can just use the class in either version of .NET.
The code also contains a real basic Windows Form, that has a folder path you can set, and a multiline textbox that will display the folder file events as they occur. You will also see how I am using the AdvancedFileSystemWatcher
to gracefully kill the watcher when the network goes down, and how the application gracefully re-creates the watcher when the network is available again. You could add code to send an email to a system admin that the network went down, but that code is for you to deal with.
I appreciate any comment or questions about this code. I wrote this quickly one morning, so it is not fully tested, and I take no responsibility that the code works flawlessly. Use at your own risk :)
Peace and happy coding.