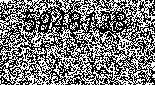
Introduction
We need to check if it is a real person who is registering on our Web site. So we decided to generate an image with a random code, hidden for robots, and feed it to the browser instead of a page response. We show it in our registration form, and let the user enter the code to check it.
Create the Web Project
- File -> New -> New project
Create a new CodeImage Visual Basic ASP.NET Web Application.
Change the Web Form
-
Rename WebForm1.aspx to CodeImage.aspx. Open it.
-
Switch to HTML View (Right click -> View HTML source).
-
Remove all HTML code. Leave only the <%@ Page ... %>
header.
-
Switch to Code View (Right click -> View Code).
Change the Page_Load
method with the code to generate the random code image. Also set Session("hiddenCode")
to allow code checks later:
Private Sub Page_Load(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles MyBase.Load
Dim ImageSrc As System.Drawing.Bitmap = _
New System.Drawing.Bitmap(155, 85)
For iX As Integer = 0 To ImageSrc.Width - 1
For iY As Integer = 0 To ImageSrc.Height - 1
If Rnd() > 0.5 Then
ImageSrc.SetPixel(iX, iY, System.Drawing.Color.White)
End If
Next iY
Next iX
Dim ImageGraphics As System.Drawing.Graphics = _
System.Drawing.Graphics.FromImage(ImageSrc)
Dim hiddenCode As String = (Fix(Rnd() * 10000000)).ToString
Session("hiddenCode") = hiddenCode
Dim drawFont As New System.Drawing.Font("Arial", _
20, FontStyle.Italic)
Dim drawBrush As New _
System.Drawing.SolidBrush(System.Drawing.Color.Black)
Dim x As Single = 5.0 + (Rnd() / 1) * (ImageSrc.Width - 120)
Dim y As Single = 5.0 + (Rnd() / 1) * (ImageSrc.Height - 30)
Dim drawFormat As New System.Drawing.StringFormat
ImageGraphics.drawString(hiddenCode, drawFont, drawBrush, _
x, y, drawFormat)
Response.ContentType = "image/jpeg"
ImageSrc.Save(Response.OutputStream, _
System.Drawing.Imaging.ImageFormat.Jpeg)
drawFont.Dispose()
drawBrush.Dispose()
ImageGraphics.Dispose()
End Sub
Build the Solution (F7), and run the application (F5).
Create the Check form.
-
Create a New Default.aspx Web Form (Right click CodeImage project -> Add -> Add Web Form).
-
Right click Default.aspx -> Set As Start Page. Open it.
-
From the IDE menu, click View -> Toolbox. From the HTML tab, drag an Image
control to the form. Right click Image
-> Properties. Set the image source to "CodeImage.aspx". Set the alternate text to "Hidden Code". Click Apply. Click OK.
-
From the HTML tab, drag a Label
control to the form. Click it twice. Change the text to "Enter the code in the image:".
-
From the Web Forms tab, drag a TextBox
control to the form.
-
From the Web Forms tab, drag a Button
control to the form.
-
From the Web Forms tab, drag a Label
control to the form. Right click -> Properties. Set the Text
property to Nothing
.
Double click the Button1
button control, and change the Button1_Click
code to:
Private Sub Button1_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles Button1.Click
If TextBox1.Text <> Session("hiddenCode") Then
Label1.Text = "Wrong Code!"
Else
Label1.Text = "Correct Code!"
End If
End Sub
Build and run the Web application (F7) (F5).
Points of Interest
That's it!
History
Just posted.