Introduction
Enums are great. They define a set of permissible values and provide a way of type-checking parameters for functions and methods. The problem is that there is a bit of gap between enums and user interfaces. So I created the RadioButtonEnum
and ListBoxEnum
controls that allow you to bind a control to an Enum
type automatically.
Background
I got fed up of building these UI components manually, and realised I should not be copying values and text from the class to the UI when the .NET framework can do this for me.
Using the code
How often do you see this in your code:
Public Enum Genders
Male
Female
End Enum
And the interface component thus:
<asp:RadioButtonList ID="RadioButtonList1" runat="server">
<asp:ListItem Value="0" Text="Male" />
<asp:ListItem Value="1" Text="Female" />
</asp:RadioButtonList>
Then the code changes, for example:
Public Enum Genders
NotSpecified
Male
Female
End Enum
Now your interface control is mismatched, and an existing value of 1 (female originally) now gets displayed as 'Male', and will throw an exception if you try to bind SelectedValue
to a 2 (Female).
Approach
I realised that it should be fairly simple to subclass a RadioButtonList
and tell it to fill itself from a given Enum
type. It actually took a bit of learning and experimenting, but it now works. In my example, I've also done this for a ListBox
control, but it can be replicated for DropDownList
s and others quite easily. To help this re-use, I have put all the code for obtaining the values from enums inside the EnumHelper
class, which can be reused from your own controls.
First, I created a subclass of the RadioButtonList
:
Public Class RadioButtonEnum
Inherits RadioButtonList
Then, I added an EnumType
property where you specify the type name of the enum. Although this worked fine for a global enum such as System.DayOfWeek
, it failed when I tried an embedded class, e.g., MyClass.MyEnum
. A few tests and a search on GetType
in MSDN revealed that enums inside classes have to be delineated with '+' and not '.', so instead the EnumType
should be specified as MyClass+MyEnum
.
When adding an enum from a module (other than the current module), you also need to specify this: e.g., if MyClass
is in TestCode.DLL which has a base namespace of XXX
, then the enum type should read: XXX.MyClass+MyEnum,TestCode
- see http://msdn2.microsoft.com/en-us/library/system.type.assemblyqualifiedname.aspx for more information.
Naming issues
Although getting the values and names for an enum type is fairly simple, the naming restrictions of enum values in code are quite tight - alphanumeric and underscores are the only permitted characters. Although an enum value of SendToCustomer
is fine in code, it's not really good practice to use this value in the user interface.
To address this, I added a FixNames
property which can be set to true to adjust enum values with underscores or CamelCase to more readable ones. Thus, SendToCustomer
becomes Send To Customer in the control.
And yet, there might be situations where we want other characters, e.g., Urgent! - or extended character sets. I therefore added the capability to look for a Description
attribute (System.ComponentModel.DescriptionAttribute
).
The actual enum value items are accessible as fields of the Enum
type, so the function GetEnumNames()
checks to see if any value has a Description
attribute and uses that value if present. E.g.:
Public Enum BookRatingWithDescription
<Description("A <b>great</b> book!")> GreatBook
<Description("Enjoyable read")> Enjoyable
<Description("Not bad - ok")> OK
Poor
<Description("Where's the shredder?")> Terrible
End Enum
HTML trick
A useful trick is that the text of the Description
attribute can contain HTML tags (this works with a RadioButtonEnum
but not a ListBoxEnum
) as shown in the first enum value above.
I also use XML a lot, so I added the ability to use XmlEnumAttribute
values if these are present, and the UseXmlNames
property is set to True
.
Example
To test this, I've included a web site that demonstrates the various functions. Here is the web page in Design mode:
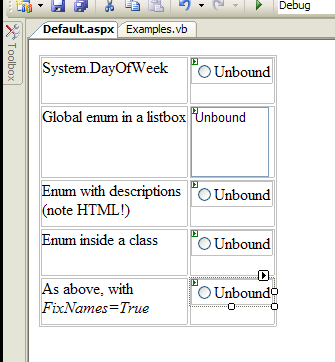
When the page is displayed, the controls are filled automatically - hurray!

In the enum with descriptions, the first item uses the HTML tags in the description. The last two examples show the same enum - in the first instance with FixNames=False
, and in the second, FixNames=True
.
Conclusion
Well, that's it - no more copying the enum values into your controls. Hope you find it useful.
History
- Version 1.0:
RadioButtonEnum
and ListBoxEnum
. Although written in VS2005, it should be possible to modify this code to work on .NET 1.x by changing the references to generic collections to supported types in 1.x.