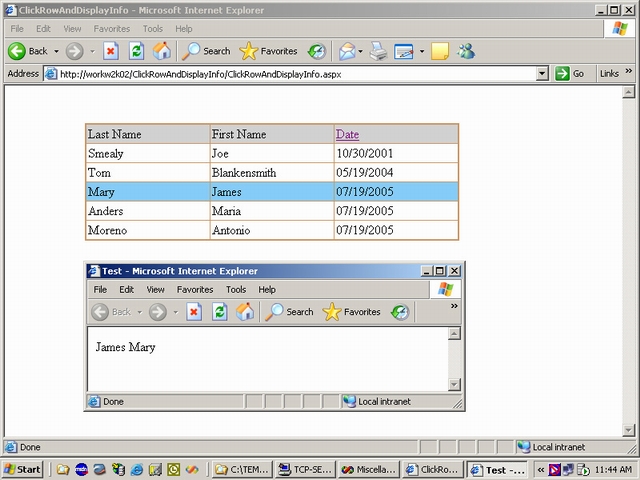
The top figure displays the highlight of the selected row. The bottom one displays the information of the selected row on the second page.
Introduction
When we click anywhere inside a row in the DataGrid, that row is highlighted and the information of the selected row will be displayed on the other page. One small disadvantage of this technique is that it adds somewhat to the stream rendered to the browser. It also adds information for each row to the view state.
Implementation
In this article, we only need to access the data item at the time it is being bound to the control, so we'll be working with the ItemDataBound
event. It is relatively easy to add alternating colors to the rows in the
DataGrid. However, when we move the mouse over the row, we may want to highlight that row and possibly add an option for a click event to the selected row. Now let's create a form before getting into the code.
<asp:datagrid id="DataGrid1"
OnItemDataBound="Item_Bound" AutoGenerateColumns="False"
CellPadding="2" SelectedItemStyle-BackColor="#33ccff"
BorderColor="#CC9966" BorderWidth="2px" EnableViewState="False"
Font-Size="12pt"
style="Z-INDEX: 101; LEFT: 100px; POSITION: absolute; TOP: 47px"
runat="server" >
<Columns>
<asp:BoundColumn DataField="LastName"
HeaderText="Last Name" ItemStyle-Width="150px">
</asp:BoundColumn>
<asp:BoundColumn DataField="FirstName"
HeaderText="First Name" ItemStyle-Width="150px">
</asp:BoundColumn>
<asp:BoundColumn DataField="Date"
HeaderText="_Date" ItemStyle-Width="150px">
</asp:BoundColumn>
<asp:ButtonColumn ButtonType="LinkButton"
CommandName="Select" Visible="False">
</asp:ButtonColumn>
</Columns>
</asp:datagrid>
Notice here that I added an OnItemDataBound
function, Item_Bound
. Add a JavaScript function to the ASPX.
<script language="javascript">
var lastColorUsed;
function DG_changeBackColor(row, highlight)
{
if (highlight)
{
row.style.cursor = "hand";
lastColorUsed = row.style.backgroundColor;
row.style.backgroundColor = '#87cefa';
}
else
row.style.backgroundColor = lastColorUsed;
}
</script>
Here is the Item_Bound
function that achieves the goals.
public void Item_Bound(object sender,
System.Web.UI.WebControls.DataGridItemEventArgs e)
{
if (e.Item.ItemType.ToString() == @"Header")
{
e.Item.BackColor = System.Drawing.Color.LightGray;
}
if ((e.Item.ItemType == ListItemType.Pager) ||
(e.Item.ItemType == ListItemType.Header) ||
(e.Item.ItemType == ListItemType.Footer))
{
return;
}
else
{
e.Item.Attributes.Add("onmouseover",
"javascript:DG_changeBackColor(this, true);");
e.Item.Attributes.Add("onmouseout",
"javascript:DG_changeBackColor(this,false);");
string strLastName =
(string)DataBinder.Eval(e.Item.DataItem, "LastName");
string strFirstName =
(string)DataBinder.Eval(e.Item.DataItem, "FirstName");
e.Item.Attributes.Add("onclick",
"javascript:DG_changeBackColor(this,true);" +
"window.open('Test.aspx?LastName=" + strLastName +
"&FirstName=" + strFirstName +
"', 'popup', 'width=600, height=200, menubar=yes,
toolbar=yes, scrollbars=yes,
titlebar=yes, resizable=yes,status=yes,status=yes ')");
}
}
I wanted to indicate how many rows the user once clicked, so I didn't de-highlight the previously clicked row.
Conclusion
As you can see, it is easy to highlight a row and display the information of the selected row on the other page. There are different methods for changing the output and formatting of the data in your DataGrid. The method you choose depends on what you want to perform.
History
- 30 July, 2007 -- Original version posted