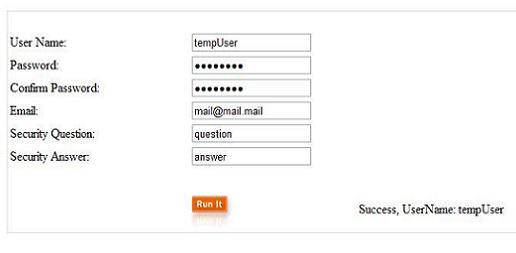
Introduction
This is a web user control to create a new user in SQL Membership in an AJAX way.
The Problem: When I click Register, the page does a postback to the server.
The Goal: To be able to click Register without loading or postback.
Background
I decided to use the Anthem .NET AJAX Framework to get the AJAX functionality because:
- You won't have to write any javascript.
- You can control the ViewState.
- You can use the Anthem controls.
Let's begin with the button. If you look at the picture (this is good :D), you can see that the 'Run it' button has two GIF images. When you click it:
- It displays another image.
- Calls a server method (asynchronously)!
- In the method, does something useful.
- Displays the original image.
But, what if I don't want to use the image button and just use a normal button? Well, this is the story of a normal button, and this is exactly what the article talks about:
- The button calls a client JavaScript function.
- This function (using Anthem) calls a server method, passing it parameters like user name, password, email, etc.
- When the server method completes, a callback comes to the client with the result calling a client JavaScript function, passing it this result object.
Using the code
Let's start with the normal button (the ASP.NET Button
control) client-side code. Don't forget to add a reference to the Anthem DLL.
Client-side code
//
//<asp:Button ID="Button2" runat="server"
// Text="Register" OnClientClick="call(); return false;"/>
//
<script type="text/javascript">
function call()
{
Anthem_InvokePageMethod(
'AddOne',
[6, 4],
CallBack(result)
);
}
function CallBack(result)
{
alert(result.value.ToString());
}
</script>
This is how we call a server method in an ASPX page: Anthem_InvokePageMethod('param1', [param2], param3(result));
. The first parameter is the method name Anthem_InvokePageMethod('Add', [param2], param3(result));
. The second parameter is the parameters you want to pass to the server method Anthem_InvokePageMethod('Add', [6, 4], param3(result));
. The third parameter is the callback client JavaScript function: Anthem_InvokePageMethod('Add', [6, 4], CallBack(result));
.
Server-side code
So, what is the server method that we want to invoke from the client-side? It's a public method in the same ASPX page with the attribute [Anthem.Method]
. So, to use it, we have to register the page to the Anthem Manager.
public partial class CreateUser : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
Anthem.Manager.Register(this);
}
[Anthem.Method]
public int Add(int param1, int param2)
{
return param1 + param2;
}
}
I think you have got it, so let's see how to do it..
Client-side code
Add HTML inputs of type text (for username, password, and email, for example) and a button.
<asp:Button ID="Button2" runat="server"
Text="Register" OnClientClick="register(); return false;"/>
<input id="NameTextInput" type="text" />
<input id="PassTextInput" type="text" />
<input id="EmailTextInput" type="text" />
<input id="resultTextInput" type="text" />
<script type="text/javascript">
function register()
{
Anthem_InvokePageMethod(
'CreateUserOnTheFly',
[document.getElementById('NameTextInput').value,
document.getElementById('PassTextInput').value,
document.getElementById('EmailTextInput').value],
function(result)
{
document.getElementById('resultTextInput').value = result.value;
}
);
}
</script>
Server-side code:
protected void Page_Load(object sender, EventArgs e)
{
Anthem.Manager.Register(this);
}
[Anthem.Method]
public string CreateUserOnTheFly(string name, string pass, string email)
{
string theReturn = "";
try
{
if (!string.IsNullOrEmpty(name))
{
MembershipCreateStatus status;
MembershipUser user = Membership.CreateUser(name, pass, email,
"question", "answer", true, out status);
#region statusIs
switch (status)
{
case MembershipCreateStatus.DuplicateEmail:
theReturn = "DuplicateEmail";
break;
case MembershipCreateStatus.DuplicateProviderUserKey:
theReturn = "DuplicateProviderUserKey";
break;
case MembershipCreateStatus.DuplicateUserName:
theReturn = "DuplicateUserName";
break;
case MembershipCreateStatus.InvalidAnswer:
theReturn = "InvalidAnswer";
break;
case MembershipCreateStatus.InvalidEmail:
theReturn = "InvalidEmail";
break;
case MembershipCreateStatus.InvalidPassword:
theReturn = "InvalidPassword";
break;
case MembershipCreateStatus.InvalidProviderUserKey:
theReturn = "InvalidProviderUserKey";
break;
case MembershipCreateStatus.InvalidQuestion:
theReturn = "InvalidQuestion";
break;
case MembershipCreateStatus.InvalidUserName:
theReturn = "InvalidUserName";
break;
case MembershipCreateStatus.ProviderError:
theReturn = "ProviderError";
break;
case MembershipCreateStatus.Success:
theReturn = "Success, User: '" + user.UserName;
break;
case MembershipCreateStatus.UserRejected:
theReturn = "UserRejected";
break;
}
#endregion
}
}
catch (Exception ex)
{
theReturn = ex.Message.ToString();
}
return theReturn;
}
Well, this is the normal button (normal function calling) code. Let's see the ImageButton
Anthem control. The ImageButton
control Click
event is a server event.
protected void ImageButton1_Click(object sender, ImageClickEventArgs e)
{
}
which brings you directly to the server-side code and handles the client-side. Then, how can we get the result? Actually, Anthem provides you with this bool
property with every Anthem control (UpdateAfterCallBack
). Then, we can do this (add an Anthem Label
control):
resultLabelAnthem.Text = theReturn;
resultLabelAnthem.UpdateAfterCallBack = true;
What you exactly do here is assign a value to the Text
property and ask the control to update itself after the callback so the client can see the assigned value. And, this is our simple control!
public partial class CreateUserAjax : System.Web.UI.UserControl
{
protected void Page_Load(object sender, EventArgs e)
{
}
protected void ImageButton1_Click(object sender, ImageClickEventArgs e)
{
string theReturn = "";
try
{
MembershipCreateStatus status;
MembershipUser user = Membership.CreateUser(TextBoxName.Text,
TextBoxPass.Text, TextBoxEmail.Text, TextBoxQuestion.Text,
TextBoxAnswer.Text, true, out status);
#region statusIs
switch (status)
{
case MembershipCreateStatus.DuplicateEmail:
theReturn = "DuplicateEmail";
break;
case MembershipCreateStatus.DuplicateProviderUserKey:
theReturn = "DuplicateProviderUserKey";
break;
case MembershipCreateStatus.DuplicateUserName:
theReturn = "DuplicateUserName";
break;
case MembershipCreateStatus.InvalidAnswer:
theReturn = "InvalidAnswer";
break;
case MembershipCreateStatus.InvalidEmail:
theReturn = "InvalidEmail";
break;
case MembershipCreateStatus.InvalidPassword:
theReturn = "InvalidPassword";
break;
case MembershipCreateStatus.InvalidProviderUserKey:
theReturn = "InvalidProviderUserKey";
break;
case MembershipCreateStatus.InvalidQuestion:
theReturn = "InvalidQuestion";
break;
case MembershipCreateStatus.InvalidUserName:
theReturn = "InvalidUserName";
break;
case MembershipCreateStatus.ProviderError:
theReturn = "ProviderError";
break;
case MembershipCreateStatus.Success:
theReturn = "Success, UserName: " + user.UserName;
break;
case MembershipCreateStatus.UserRejected:
theReturn = "UserRejected";
break;
}
#endregion
}
catch (Exception ex)
{
theReturn = ex.Message.ToString();
}
resultLabelAnthem.Text = theReturn;
resultLabelAnthem.UpdateAfterCallBack = true;
}
}
Points of interest
I think it is very simple invoking server methods asynchronously, and callbacks are the way to put your code in an AJAX way. Well, not every time! I tried to make an AJAX File Uploader control, added many scripts, ActiveX.. but finally, the security system stopped the stream to read the files on the client computer and I noticed I was trying to upload files from the client without his permission, asynchronously in an AJAX way! Some developers say they did it with the FileUpload
control and iFrames.. I tried that, but I failed. Please tell me about your experience.