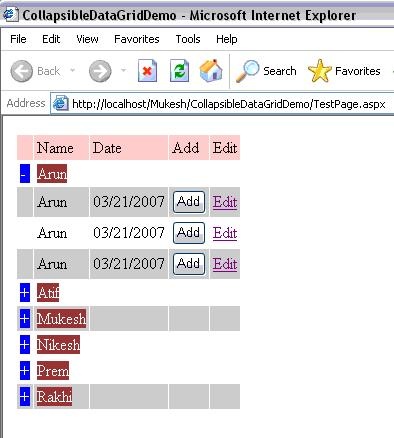
Introduction
Sometimes it becomes very difficult for beginners in ASP.NET to incorporate JavaScript functions with their server side controls. They know what they want to do, but they are stuck on how to do it. This happened to me when I had to make a DataGrid collapsible and expandable on a sorted column. I could do that with a nested DataGrid, but the drawback would be postback on every click. So I made a library which could convert any ASP.NET DataGrid (or GridView in ASP.NET 2.0) into a client-side collapsible DataGrid on a sorted column of my DataSet.
How to Use CollapsibleDatagrid.dll
The second link given above is for CollapsibleDataGrid.dll and the JavaScript file CollapsibleGrid.js, and the first one is for the sample demo project made using this CollapsibleDataGrid.dll and CollapsibleGrid.js.
In the second link, you will get the following:

Here you will find another DLL, CollasibleGridView.dll.This is the same as CollapsibleDataGrid.dll but can be used if you are using the ASP.NET 2.0 GridView
. By going through the following section, you can easily understand how to use my CollapsibleDataGrid.dll to convert your ASP.NET DataGrid
into a collapsible DataGrid
.
Open Visual Studio .NET and open a new ASP.NET project CollapsibleDataGridDemo. Delete the default webpage and add a new web page TestPage.aspx. Now right click your project in Solution Explorer and add a reference to CollapsibleDataGrid.dll.


Now add a the JavaScript file Collasiblegrid.js to your project by right clicking your project and using the Add Existing Item option.

Now to add the JavaScript, add this code to the <head>
tag of your TestPage.aspx page:
<script type="text/javascript" src="CollapsibleGrid.js">
This JavaScript file contains the following code:
function ShowHideData(obj,crl_names,ColpsText,ExpText)
{
try
{
var RowIds=crl_names.split(",");
var val=obj.innerHTML;
if (val==ExpText)
{
for (var i=0;i<RowIds.length-1;i++)
{
document.getElementById(RowIds[i]).style.display = "block";
}
obj.innerHTML=ColpsText;
}
else
{
for (var i=0;i<RowIds.length-1;i++)
{
document.getElementById(RowIds[i]).style.display = "none";
}
obj.innerHTML=ExpText;
}
}
catch(err)
{
alert(err);
}
}
function CollapseAllData(crl_names)
{
if (crl_names!="")
{
var RowIds=crl_names.split(",");
for (var i=0;i<RowIds.length-1;i++)
{
document.getElementById(RowIds[i]).click();
}
}
}
Now, copy the following .aspx code in TestPage.aspx, which includes an ASP.NET DataGrid
control, and now we want to make it collapsible on the Names column, which is already sorted.
<body MS_POSITIONING="GridLayout">
<form id="Form1" method="post" runat="server">
<table id="table1">
<tr>
<td>
<asp:datagrid id="DgCollapsible" runat="server"
AutoGenerateColumns="false" BorderWidth="0" CellSpacing="1"
CellPadding="3" ShowFooter="True"
BorderStyle="None" Width="100%" AllowPaging="false">
<AlternatingItemStyle BorderWidth="1px" BorderStyle="Ridge"
BorderColor="#404040" BackColor="#E0E0E0"></AlternatingItemStyle>
<ItemStyle></ItemStyle>
<HeaderStyle BackColor="#FFE0C0"></HeaderStyle>
<FooterStyle></FooterStyle>
<Columns>
<asp:TemplateColumn>
<ItemTemplate>
<asp:Label Width="10px" runat="server" ID="ClickableCol"></asp:Label>
</ItemTemplate>
</asp:TemplateColumn>
<asp:TemplateColumn HeaderText="Name">
<ItemTemplate>
<asp:Label runat="server" ID="NamesCol">
<%#DataBinder.Eval(Container, "DataItem.Names").ToString()%>
</asp:Label>
</ItemTemplate>
</asp:TemplateColumn>
<asp:BoundColumn DataField="Dates" HeaderText="Date"></asp:BoundColumn>
<asp:TemplateColumn HeaderText="Add">
<ItemTemplate>
<asp:Button Runat="server" ID="btnCommand" Text="Add"></asp:Button>
</ItemTemplate>
</asp:TemplateColumn>
<asp:TemplateColumn HeaderText="Edit">
<ItemTemplate>
<a href="#" runat="server" id="linkCommand">Edit</a>
</ItemTemplate>
</asp:TemplateColumn>
</Columns>
</asp:datagrid>
</td>
</tr>
</table>
</form>
</body>
Now you have to write the sever side code for making your DataGrid
collapsible.
Protected Function GetDataSetForCollapsibleDataGrid() As DataSet
Dim ds As DataSet = New DataSet
ds.Tables.Add("Main")
Dim arrDates() As String = {"03/21/2007", "03/21/2007", _
"03/21/2007", "04/10/2007", "05/11/2007", _
"05/11/2007", "06/11/2007", "04/10/2007", _
"05/11/2007", "05/11/2007", "03/21/2007", _
"04/10/2007", "05/11/2007", "05/11/2007", _
"06/11/2007", "04/10/2007", "05/11/2007", _
"05/11/2007", "05/11/2007", "05/11/2007"}
Dim arrNames() As String = {"Arun", "Arun", "Arun", _
"Atif", "Atif", "Atif", "Atif", _
"Mukesh", "Mukesh", "Nikesh", "Prem", _
"Prem", "Prem", "Prem", "Rakhi", _
"Rakhi", "Rakhi", "Rakhi", "Rakhi", "Rakhi"}
ds.Tables(0).Columns.Add("Dates")
ds.Tables(0).Columns.Add("Names")
Dim dr As DataRow
Dim i As Integer = 0
Do While (i < arrDates.Length)
dr = ds.Tables(0).NewRow
dr(0) = arrDates(i)
dr(1) = arrNames(i)
ds.Tables(0).Rows.Add(dr)
i = i + 1
Loop
Return ds
End Function
Here, GetDataSetForCollapsibleDataGrid()
function creates the DataSet
sorted by names. In an actual scenario, this can be obtained from the database. For a collapsible DataGrid
, you need to have your DataSet
sorted on any field you want to make collapsible. Here, the DataSet
contains names like Arun, Mukesh, Nikesh, Prem, and Rakhi in a sorted way, and now we will make a DataGrid
collapsible on these name, means same name records will be together and can be collapsed and expanded on a click. For details see the image on the top.
After having your DataSet
ready, you can use the CollapsibleDatagrid
class.
Private Sub BindCollpsibleGrid()
Dim ds As DataSet = GetDataSetForCollapsibleDataGrid()
Dim objCollapsibleDataGrid As New _
CustomWebControl.CollapsibleDataGrid(DgCollapsible)
objCollapsibleDataGrid.DataSource = ds
objCollapsibleDataGrid.SortByFieldName = "Names"
objCollapsibleDataGrid.SortOnLength = 10
objCollapsibleDataGrid.SortByColumnID = "NamesCol"
objCollapsibleDataGrid.SortByCellBackColor = Drawing.Color.Brown
objCollapsibleDataGrid.SortByCellForeColor = Drawing.Color.White
objCollapsibleDataGrid.ClickableColumnID = "ClickableCol"
objCollapsibleDataGrid.ClickableCellBackColor = Drawing.Color.Blue
objCollapsibleDataGrid.ClickableCellForeColor = Drawing.Color.White
objCollapsibleDataGrid.Style = _
CustomWebControl.CollapsibleDataGridStyle.ExpandOnlyFirstRow
objCollapsibleDataGrid.ExpandableText = "+"
objCollapsibleDataGrid.CollapsibleText = "-"
objCollapsibleDataGrid.Bind()
End Sub
In the BindCollpsableGrid()
function, we create an object of CollapsibleDataGrid
: objCollapsibleDataGrid as new CustomWebControl.CollapsibleDataGrid(DgCollapsible)
. Here, the CollapsibleDataGrid
constructor takes the original DataGrid
, in this case, DgCollapsible
, as the parameter.
There are number of properties of CollapsibleDataGrid
you need to set. Some are mandatory, some are optional.
After creating the CollapsibleDataGrid
object, you need to set your sorted DataSet
as the DataSource
. SortByFieldName
is nothing but the field name by which your DataSet
is sorted and you want to make it collapsible. SortByColumnID
is the column ID of your DataGrid
to which the sorted column of your DataSet
is bound. ClickableColumnID
is the column ID (should be the first column) of your DataGrid
which you want to make clickable for collapsing and expanding rows.
There is a property SortOnLength
which is the length for which your sorted column values will be checked for making groups. Let's say, your sorted column is a date time, but you want the collapsing to work only on some dates, so you need to set the length as 10 (if date format is 10-12-2007 15:12:00), or as the length of the date part. If nothing is assigned, then the whole value of the column will be checked such as Names here.
Other properties include SortByCellBackColor
, SortByCellForeColor
, ClickableCellBackColor
, ClickableCellForeColor
, ExpandableText
(by default, this is "+"), and CollapsibleText
(by default, this is "-").
Here is the complete server-side code for TestPage.aspx:
Public Class TestPage
Inherits System.Web.UI.Page
#Region " Web Form Designer Generated Code "
<System.Diagnostics.DebuggerStepThrough()> Private Sub InitializeComponent()
End Sub
Protected WithEvents DgCollapsable As System.Web.UI.WebControls.DataGrid
Protected WithEvents DgCollapsible As System.Web.UI.WebControls.DataGrid
Private designerPlaceholderDeclaration As System.Object
Private Sub Page_Init(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles MyBase.Init
InitializeComponent()
End Sub
#End Region
Private Sub Page_Load(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles MyBase.Load
BindCollpsibleGrid()
End Sub
Protected Function GetDataSetForCollapsibleDataGrid() As DataSet
Dim ds As DataSet = New DataSet
ds.Tables.Add("Main")
Dim arrDates() As String = {"03/21/2007", "03/21/2007", _
"03/21/2007", "04/10/2007", "05/11/2007", _
"05/11/2007", "06/11/2007", "04/10/2007", _
"05/11/2007", "05/11/2007", "03/21/2007", _
"04/10/2007", "05/11/2007", "05/11/2007", _
"06/11/2007", "04/10/2007", "05/11/2007", _
"05/11/2007", "05/11/2007", "05/11/2007"}
Dim arrNames() As String = {"Arun", "Arun", "Arun", _
"Atif", "Atif", "Atif", "Atif", _
"Mukesh", "Mukesh", "Nikesh", "Prem", _
"Prem", "Prem", "Prem", "Rakhi", _
"Rakhi", "Rakhi", "Rakhi", "Rakhi", "Rakhi"}
ds.Tables(0).Columns.Add("Dates")
ds.Tables(0).Columns.Add("Names")
Dim dr As DataRow
Dim i As Integer = 0
Do While (i < arrDates.Length)
dr = ds.Tables(0).NewRow
dr(0) = arrDates(i)
dr(1) = arrNames(i)
ds.Tables(0).Rows.Add(dr)
i = i + 1
Loop
Return ds
End Function
Private Sub BindCollpsibleGrid()
Dim ds As DataSet = GetDataSetForCollapsibleDataGrid()
Dim objCollapsibleDataGrid As New _
CustomWebControl.CollapsibleDataGrid(DgCollapsible)
objCollapsibleDataGrid.DataSource = ds
objCollapsibleDataGrid.SortByFieldName = "Names"
objCollapsibleDataGrid.SortOnLength = 10
objCollapsibleDataGrid.SortByColumnID = "NamesCol"
objCollapsibleDataGrid.SortByCellBackColor = Drawing.Color.Brown
objCollapsibleDataGrid.SortByCellForeColor = Drawing.Color.White
objCollapsibleDataGrid.ClickableColumnID = "ClickableCol"
objCollapsibleDataGrid.ClickableCellBackColor = Drawing.Color.Blue
objCollapsibleDataGrid.ClickableCellForeColor = Drawing.Color.White
objCollapsibleDataGrid.Style = _
CustomWebControl.CollapsibleDataGridStyle.ExpandOnlyFirstRow
objCollapsibleDataGrid.ExpandableText = "+"
objCollapsibleDataGrid.CollapsibleText = "-"
objCollapsibleDataGrid.Bind()
End Sub
End Class
Points of Interest
To use collasibleDataGrid.dll, your DataSet
should be sorted on a particular column (in my demo project, I used the Names field) on which the DataGrid
will be collapsed or expanded. Do not forget to add a template column of ASP.NET Label
with ID at the top of the columns of your DataGrid
.
You can achieve the same results for ASP.NET GridView
using CollasibleGridView.dll and the same JavaScript file.
History
- First posted on 04-Dec-2007.
- Updated on 21-Dec-2007.