Introduction
ASP.NET AJAX Extender controls add features (usually AJAX or JavaScript support) to existing controls already declared on a page. With VS 2005, you had to manually wire-up control extenders yourself (either via source-view or via the property grid). This is not necessary with the newly released VS 2008, and can be done easily using the "Add Extender" feature. The following example demonstrates the addition of AJAX support to a TextBox
in VS 2008.
Using the code
Step 1: Create a simple ASP.NET website using the VS 2008 templates by accepting defaults at a suitable location.
Step 2: Add a new project using File->Add->New Project->Visual C# -> ASP.NET AJAX Server Control Extender, as shown:
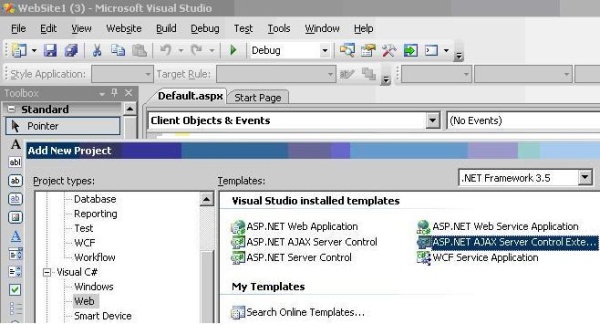
Step 3: Modify ExtenderControl1.cs as follows. Add the following attributes to the ExtenderControl1
class:
[
TargetControlType(typeof(TextBox))
]
[
DefaultProperty("Contacts"),
ParseChildren(true, "Contacts"),
ToolboxData(
"<{0}:QuickContacts runat=\"server\"> </{0}:QuickContacts>")
]
Step 4: Add a property as follows to the ExtenderControl1
class:
private ArrayList contactsList;
[
Category("Behavior"),
Description("The contacts collection"),
DesignerSerializationVisibility(
DesignerSerializationVisibility.Content),
Editor(typeof(ContactCollectionEditor), typeof(UITypeEditor)),
PersistenceMode(PersistenceMode.InnerDefaultProperty)
]
public ArrayList Contacts
{
get
{
if (contactsList == null)
{
contactsList = new ArrayList();
}
return contactsList;
}
}
Step 5: Modify the GetScriptDescriptors
method as follows:
protected override IEnumerable<ScriptDescriptor>
GetScriptDescriptors(System.Web.UI.Control targetControl)
{
ScriptBehaviorDescriptor descriptor = new ScriptBehaviorDescriptor(
"AjaxTextWithTableExtender.ClientBehavior1",
targetControl.ClientID);
descriptor.AddProperty("contactsList", this.Contacts);
return new ScriptBehaviorDescriptor[] { descriptor };
}
Step 6: Modify the ClientBehavior1.initializeBase
function by adding the following lines:
this.contactsList=null;
this.strtext=null;
this.flag=true;
Add the following lines to ClientBehavior1.prototype
Initialize
function. Note: ve careful with the commas.
$addHandler(this.get_element(), 'keyup',
Function.createDelegate(this, this._onkeyup));
this._onkeyup();
and also add the following:
get_contactsList : function() {
return this.contactsList;
},
set_contactsList : function(value) {
this.contactsList=value;
this.raisePropertyChanged('contactsList');
},
_onkeyup : function() {
this.strtext=document.getElementById("TextBox1").value;
var links = new Array ("link1", "link2", "link3");
var links_url = new Array ("link1.htm", "link2.htm",
"link3.htm");
var loc=String(this.location);
loc=loc.split("/");
loc=loc[loc.length-1].split(".");
loc=loc[loc.length-2];
var v=new Array(this.contactsList);
var t = document.getElementById("TextBox1");
if (t) {
if(this.flag)
{
var mybody = document.getElementsByTagName("body")[0];
mytable = document.createElement("table");
mytablebody = document.createElement("tbody");
for(var j = 0; j < 1; j++) {
for(var i = 0; i < 2; i++) {
mycurrent_row = document.createElement("tr");
if(this.strtext!=null & v[0][i].Email.substring(0,1)!=null)
{
if(v[0][i].Email.substring(0,1)==this.strtext.substring(0,1))
{
mycurrent_cell = document.createElement("td");
linkEmail=document.createElement("a");
linkEmail.setAttribute("href",links_url[0]);
mycurrent_cell.appendChild(linkEmail);
var stre=v[0][i].Email;
currenttext = document.createTextNode(stre);
linkEmail.appendChild(currenttext);
mycurrent_row.appendChild(mycurrent_cell);
mycurrent_cell = document.createElement("td");
stre=v[0][i].Name;
currenttext = document.createTextNode(stre);
mycurrent_cell.appendChild(currenttext);
mycurrent_row.appendChild(mycurrent_cell);
mycurrent_cell = document.createElement("td");
var stre=v[0][i].Phone;
currenttext = document.createTextNode(stre);
mycurrent_cell.appendChild(currenttext);
mycurrent_row.appendChild(mycurrent_cell);
}
}
mytablebody.appendChild(mycurrent_row);
}
}
mytable.appendChild(mytablebody);
mybody.appendChild(mytable);
mytable.setAttribute("border","2");
if(this.strtext=='')
this.flag=true;
else
this.flag=false;
}
}
}
Step 7: The editor used to create the contacts collection is ContactCollectionEditor, which is based on the example found at: http://msdn2.microsoft.com/en-us/library/ms178654.aspx. Now compile the ExtenderControl project. Open the designer of your website's Default.aspx. You will find your ExtenderControl added at the top of the Toolbox. Now add a Label
and a TextBox
as shown:

Step 8: Click on the arrow to the right of the TextBox
and choose "Add Extender". The following screen pops up:

Step 9: Select ExtenderControl1
and accept the default ID for the extender. If everything is fine, a "Remove Extender" option will appear below the "Add Extender" option for the TextBox
. Right click the TextBox
and in Properties, navigate to TextBox1_Extender
. Add some dummy records to the Contacts collection with the following ContactCollectionEditor:

Step 10: Add an HTML file called "link1.htm" to your website project. Build the example and run the website.
Test the control
Add the letter 'a' or the first letter of one of the email records you entered, and watch the extender control work. It should be something like this:

The matching records from the Contacts collection are displayed and they can be linked as well.