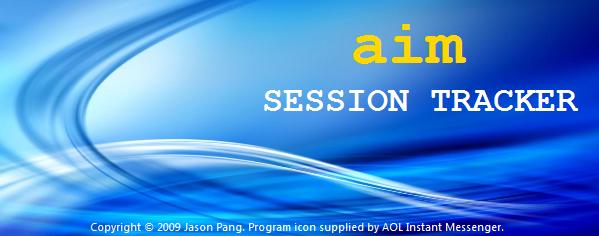

Introduction
This is my first CodeProject article. I've made a small AIM instant messenger session tracker utility. I took the image from here. If this is a copyright violation, please let me know.
This program polls the status of any AIM user by visiting a special link.
The Code
The essence of the program is only one line:
http://api.oscar.aol.com/presence/get?k=DEVELOPERKEY&f=xml&t=SCREENNAME1&t=SCREENNAME2...
This allows you to get the state of the AIM user - online, away, offline. Note that it is not possible to check if a user is invisible or not using this URL (although there is a way to check if a user was invisible or not after they come back online). Also, you need a developer key to use this service (you can get one here).
Since this URL returns an XML document, I processed it with XML tools from the C# library. Here's the general method I used to get each individual element; you can't copy and paste it into Visual Studio, but it should give you a general direction:
XmlDocument xDoc = new XmlDocument();
xDoc.Load("http://api.oscar.aol.com/presence/get?k=DEVELOPERKEY&f=xml&t=SCREENNAME");
XmlNodeList Users = xDoc.GetElementsByTagName("user");
foreach (XmlNode User in Users)
{
string Screenname = User["displayId"].InnerText;
}
Points of Interest
I've always noticed .NET programs take up a fair chunk of memory, so I Googled 30 minutes about how to reduce .NET program memory sizes. And, I found a useful link: http://bytes.com/groups/net-c/302104-forcefully-releasing-memory.
This is how to reduce program memory size:
public static void ReduceMemoryUsage()
{
try
{
Process CurrentProcess = Process.GetCurrentProcess();
CurrentProcess.MaxWorkingSet = CurrentProcess.MinWorkingSet;
}
catch { }
}
Conclusion
There's not much to the code. Download and use the program as it is. The developer key in the download above is my developer key, so I would appreciate it if each of you could get your own developer key.