Introduction
This application makes simple client side form validation with the help of jQuery plugins. Here I would like to show different validation style of jQuery with basic sample of HTML and jQuery.
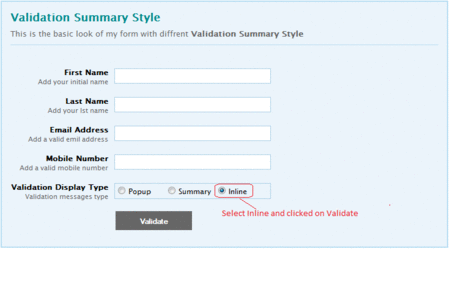
I have published article at http://saffroze.com/jquery-validation-styles/
Background
ASP.NET MVC 3 uses jQuery validation for form validations and jQuery validation library has a remote validation feature, using this feature by ASP.NET MVC libraries is really easy you can find more about it http://docs.jquery.com/Plugins/validation.
- Every validation attribute has a ToJson method which serializes attributes into a
dictionary
$("#form").validate({
rules: {
firstname: { required: true, minlength: 5, maxlength: 10 },
lastname: { required: true, minlength: 5, maxlength: 10 },
email: { required: true, email: true },
mobile: { required: true, digits: true, minlength: 10, maxlength: 10 }
}
});
- All error labels are displayed inside an unordered list with the ID
“validationSummary” Additonal container for error messages. The elements
given as the “errorContainer” are all shown and hidden when errors
occur.
errorContainer: "#validationSummary"
- The error labels themselve are added to the element(s) given as errorLabelContainer, here an unordered list.
errorLabelContainer: "#validationSummary ul"
- Therefore the error labels are also wrapped into li elements (wrapper option).
wrapper: “li” - A custom message display handler. Gets the map of errors as the first
argument and and array of errors as the second, called in the context of
the validator object.
showErrors: function (errorMap, errorList) {
.
.
.
}
Implementation
This code demonstrates a basic jQuery validation sample, jQuery Validate
plugins validates the form before it submitted, if form is valid it
submits the form, otherwise form doesn’t get submitted.
- Include jQuery Plugin and Validations scripts into your page.
<script type="text/javascript" src="../../Scripts/jquery.validate.js"></script>
<script type="text/javascript" src="../../Scripts/jquery-ui-1.8.11.js"></script>
- Add following script in your page. It is very descriptive so no need to explain each and every lines.
$(document).ready(function () {
$('.text')
.focus(function () { $('.text').removeClass('focused'); $(this).addClass('focused'); })
.blur(function () { $(this).removeClass('focused'); });
$("#form").validate({
rules: {
firstname: { required: true, minlength: 5, maxlength: 10 },
lastname: { required: true, minlength: 5, maxlength: 10 },
email: { required: true, email: true },
mobile: { required: true, digits: true, minlength: 10, maxlength: 10 }
},
errorContainer: "#validationSummary",
errorLabelContainer: "#validationSummary ul",
wrapper: "li",
showErrors: function (errorMap, errorList) {
$('.errorList').hide();
$('.inlineMessage').hide();
$('#validationSummary').hide();
var messages = "";
$.each(errorList, function (index, value) {
var id = $(value.element).attr('id');
messages += "<span>" + (index + 1) + ". <a title='click to view field' href='javascript:setFocus(" + id + ");'>[" + $(value.element).attr('name') + "]</a> " + value.message + "</span>
";
});
messages = "
<div class='errorWrapper'>
<h1>Please correct following errors:</h1>
" + messages + "</div>
";
switch ($('input[name=DisplayType]:checked', '#form').val()) {
case "Popup":
$('#dialog-validation').html(messages);
$('#dialog-validation').dialog('open');
break;
case "Summary List":
$('#summary-validation').html(messages);
$('#summary-validation').show("fast");
break;
case "Inline":
$.each(errorList, function (index, item) {
$(item.element).next('div').html("<span style='font-weight:bold'>[" + $(item.element).attr('name') + "]</span> " + item.message);
$(item.element).next('div').show("fast");
});
break;
}
},
submitHandler: function () {
$('#summary-validation').empty();
$('#dialog-validation').empty();
alert("All fields are valid!")
}
})
$("#dialog-validation").dialog({
resizable: false,
height: 190,
width: 350,
modal: true,
autoOpen: false,
title: "Validation Errors",
buttons: {
"Ok": function () {
$(this).dialog("close");
$('.focused').focus();
}
}
});
});
function setFocus(ele) {
$(ele).focus();
}
- HTML page:
- If you need show validation near to field then it should be compulsory to add “” with class name is “inlineMessage”.
<div class="formWrapper" id="formStyle"><form id="form" action="" method="post" name="form"><label>First Name</label> <input id="firstname" class="text" type="text" name="firstname" />
<div class="inlineMessage"></div> </form>
</div>
- Hidden container for Error Messages
<div id="validationSummary">
<ul class="errorMessageList">
</ul>
</div>
- Display Validation Summary in same page
<div style="display: none;" id="summary-validation" class="errorList">
</div>
- Display Validation Summary in Modal Dialog box.
<div style="display: none;" id="dialog-validation" class="errorList">
</div>
- Add Style for Form, Inputbox, Button, Dialog and Validation Messages.Please visit Views/Shared/_Layout.cshtml

Your Thoughts
If you find some issues or bugs with it, just leave a comment or drop me an email. If you make any notes on this, let me know that too so I don’t have to redo any of your hard work. Please provide a
“Vote” if this would be helpful.