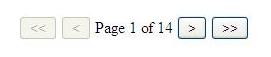
Introduction
The Repeater
and DataList
are two very important controls and many developers use at least one of them in most of their websites or web applications. Also, these Controls are easy to use for UI developers, but their features are limited. The Gridview control has a very useful paging feature, but it's a complex control and you might not want to use it. And, if you want to use a Repeater
or a DataList
, they do not support paging, so what will you do ?!!
I will show you how to make a Repeater
or a DataList
that allows paging. I'll also make a web user control that anyone can use to allow paging in a Repeater
or DataList
control.
How to Decide to Use the DataGrid, DataList, or Repeater
The URL below describes the differences between a DataGrid
, DataList
, and a Repeater
and helps to decide which one to use: http://msdn.microsoft.com/en-us/library/aa479015.aspx.
Advantages of the AllowPaging Control
It enables paging in Repeater
and DataList
controls. It is an easy to use Web User Control. The control depends on ObjectDataSource
so, if you want to use SqlDataSource
, just replace ObjectDataSource
with SqlDataSource
.
Using the code
The sample code is divided into three parts:
- The Default Page to add a
Repeater
or DataList
control. - The
UCPager
Web User Control that is responsible for paging. - The
GetData
class to get data from the database.
Also, I'm using the Northwind database in this sample.
First, I'll talk about the User Control sample code. There are four important properties:
CurrentPage
- sets or gets the current page numberOds
- pass to it the ObjectDataSource
ObjectControl
- pass a Repeater
or DataList
PageSize
- pass an integer for how many records will appear in each page
public int CurrentPage
{
get
{
object obj = this.ViewState["_CurrentPage"];
if (obj == null)
{
return 0;
}
else
{
return (int)obj;
}
}
set
{
this.ViewState["_CurrentPage"] = value;
}
}
public ObjectDataSource Ods { get; set; }
public object ObjectControl { get; set; }
public int PageSize { get; set; }
The method below is responsible for binding a control and enabling or disabling the navigation buttons depending on how records are shown.
private int GetItems()
{
PagedDataSource objPds = new PagedDataSource();
objPds.PageSize = PageSize;
objPds.DataSource = Ods.Select();
objPds.AllowPaging = true;
int count = objPds.PageCount;
objPds.CurrentPageIndex = CurrentPage;
if (objPds.Count > 0)
{
btnPrevious.Visible = true;
btnNext.Visible = true;
btnLastRecord.Visible = true;
btnFirstRecord.Visible = true;
lblCurrentPage.Visible = true;
lblCurrentPage.Text = "Page " +
Convert.ToString(CurrentPage + 1) + " of " +
Convert.ToString(objPds.PageCount);
}
else
{
btnPrevious.Visible = false;
btnNext.Visible = false;
btnLastRecord.Visible = false;
btnFirstRecord.Visible = false;
lblCurrentPage.Visible = false;
}
btnPrevious.Enabled = !objPds.IsFirstPage;
btnNext.Enabled = !objPds.IsLastPage;
btnLastRecord.Enabled = !objPds.IsLastPage;
btnFirstRecord.Enabled = !objPds.IsFirstPage;
if (ObjectControl is DataList)
{
DList = (DataList)ObjectControl;
DList.DataSource = objPds;
DList.DataBind();
}
else if (ObjectControl is Repeater)
{
Rep = (Repeater)ObjectControl;
Rep.DataSource = objPds;
Rep.DataBind();
}
return count;
}
In the First Record button:
CurrentPage = 0;
GetItems();
In the Last Record button:
CurrentPage = GetItems() -1;
GetItems();
In the Previous Record button:
CurrentPage -= 1;
GetItems();
In the Next Record button:
CurrentPage += 1;
GetItems();
In the Default Page, all you need is bind the AllowPaging
control properties, and the code below explains that:
UCPager1.Ods = ObjEmployees;
UCPager1.ObjectControl = Repeater1;
UCPager1.PageSize = 1;
I'm waiting for your comments. You can read my blog here: http://waleedelkot.blogspot.com/.
Points of Interest
This control saves a lot of time for me, and I hope it helps you too.
History
- 27-08-2009: First version released.