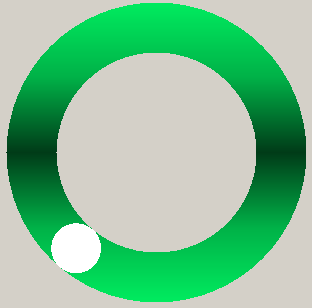
Introduction
If you have been looking for an indeterminate progress indicator control for a WinForms application, this may be useful. It is modeled on the relatively new GoogleTM progress indicator (an annulus with a rotating indicator). The control has properties to change its color, size, rotation speed, and type (externally pulsed or animated).
Background
Although DirectX, XNA, and WPF have gained acceptance, the development of this control was limited to Drawing2D. Many users of WinForms may not have the prerequisites for any of the more advanced methods.
Using the Code
The run-time view of this control is controlled by the following properties and methods.
Properties
Name | Type | Units | Default | Purpose |
Animate | bool | Boolean | true | Starts (true) and stops (false) the indicator animation |
AnnulusColor | Color | Color | Pale Turquoise | Sets or gets the base color of the annulus |
BackgroundColor | Color | Color | SystemColor.
Control | Sets or gets the background color of the control |
IndicatorAngularAdvance | int | Degrees | 10 | Sets or gets the number of degrees that the indicator moves on each tick or pulse |
IndicatorColor | Color | Color | White | Sets or gets the color of the rotating indicator |
IndicatorType | enum | INDICATORTYPES | ANIMATED | Sets or gets whether the control is ANIMATED or PULSED |
InnerRadius | int | Pixels | 8 | Sets or gets the radius of the annulus inner circle |
OuterRadius | int | Pixels | 12 | Sets or gets the radius of the annulus outer circle |
RefreshRate | int | Milliseconds | 150 | Sets or gets the timer tick interval (i.e., the time between control refreshes) |
TransitionColor | Color | Color | Gray | Sets or gets the transition color at annulus mid-position |
Methods
Name | Result |
Pulse | Causes indicator to advance |
Dimensions and Color Effects

The control dimensions are determined by the OuterRadius (or) value. In the preceding figure, the outer rectangle (red) is the control client rectangle.
The effect of a gradually changing color is called a "blend". In this case, the effect is obtained by using a two-color linear gradient brush along a vertical with a linear blend from the starting color (AnnulusColor) to the ending color (TransitionColor). The blend pattern is specified by two equally sized arrays, named "positions" and "factors". Both arrays contain proportions. The positions array contains values from 0.0F to 1.0F that define proportional distances along the vertical. The factors array contains values from 0.0F to 1.0F that define proportional color from the starting to the ending color along the vertical. In the preceding figure, the values in parentheses are the positions and factors, respectively, for blending of the linear gradient brush.
The InnerRadius (ir) value determines the circle (actually ellipse) that will be excluded from the center of the control. The difference between the outer radius and the inner radius values is the annulus thickness. The annular thickness is also the diameter of the rotating indicator.
Building the Control
The control is built in sixteen steps.

Both the control's client rectangle and the outer bounding rectangle are specified by the value of OuterRadius. In step two, the background is colored as BackgroundColor. Then the outer bounding rectangle is used to develop the outer region, first by creating a path from an ellipse and then by creating a region from the path. The inner bounding rectangle is specified by the value of InnerRadius and is used to develop the inner region. Regions are used to define the annulus by excluding the inner region from the outer region (step 8). Color is applied using a Linear Gradient Brush with a starting color of AnnulusColor and an ending color of TransitionColor. Blending is performed as described earlier. The annulus is built in its own graphics buffer and is only revised when a color- or size-changing event occurs.
The indicator is built in its own graphic buffer at every timer tick or whenever the control is pulsed. The indicator is initially drawn at the right center position of the annulus. Then a translation and a rotation transformation occur. Thereafter both graphics (annulus and indicator) are rendered to the screen.
Control Source Code
The source code was developed using the Microsoft Visual C# 2008 Express Edition. In the event that the reader downloads this development environment, the MSDN Help Library for the Visual C# 2008 Express Edition should also be downloaded. The source code in GoogleProgressIndicator.cs contains the source for the GraphicsBuffer
class. The two classes appear in the same compilation unit so that a reference to an external class (i.e., GraphicsBuffer
) is not required.