NOTE: If you are using Visual Studio use the Packet Manager NuGet to obtain the library: OSIcon
If you want to obtain the most recent source code, contribute, fork or open an issue, please go to github OSIcon project.
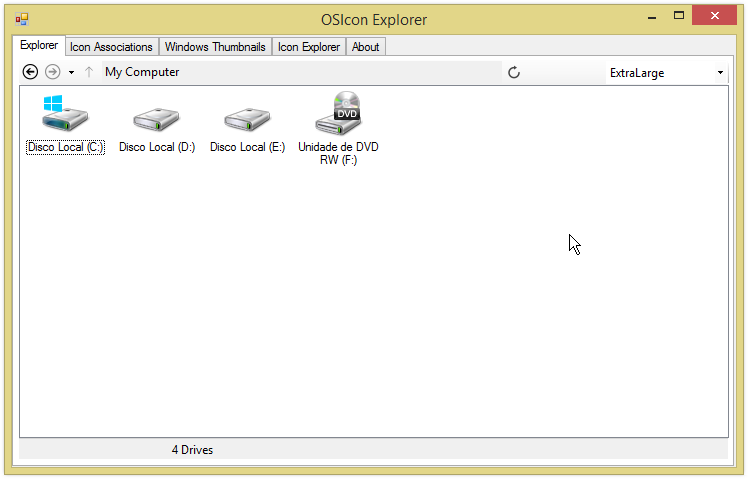






Table of Contents
This library can retrieve icons from extensions and files, with additional information like file type (hard drive, folder, PHP Script File, ...). The library also provides a class for CACHE icons and creates an ImageList
with the added icons.
WinAPI
namespace (Contains all Win API calls)
Shell32 static
class (all API calls for Shell32.dll) - DwmApi static class (all API calls for Dmwapi.dll)
User32 static
class (all API calls for User32.dll) Comctl32 static
class (all API calls for Comctl32.dll) IShellFolder
interface
-
<span style="color: rgb(153, 0, 0); font-family: Consolas, 'Courier New', Courier, mono; font-size: 14.6666669845581px;">Controls</span>
FileExplorer
A control that behaves like Windows Explorer
- Utilities static class (helper functions)
IconReader static
class (read, handle icons) IconManager
class (IconReader
wrapper with caching features, automatic creation of ImageList
) WindowsThumbnail
class to retrieve Windows thumbnails on taskbar About static
class (stores some constants about the library, author, webpage, etc.)
Get any icon from a file using "OSIcon.IconReader.ExtractIconFromFile(path, isLarge);
".
Icon icon = OSIcon.IconReader.ExtractIconFromFile("C:\\pathtofile.png", true);
Now, if you want to extract an icon from a resource file like shell32.dll, you can do: "OSIcon.IconReader.ExtractIconFromFile(path, iconIndex);
".
Icon icon = OSIcon.IconReader.ExtractIconFromFile("C:\\Windows\\system32\\shell32.dll", 5);
In Windows Explorer, FileZilla, etc., we can see the file type (Folder, PHP Script, Dynamic Link Library, etc.). To retrieve that information, we need to use some API calls. Using OSIcon, this is quite simple: "OSIcon.IconReader.GetFileIcon(pathOrExtension, IconReader.IconSize);
".
IconInfo iconInfo = OSIcon.IconReader.GetFileIcon(".png", IconReader.IconSize.Large);
MessageBox.Show(string.Format("Display Name: {0}\nFile Type: {1}",
iconInfo.DisplayName, iconInfo.TypeName));
We can also retrieve file extensions from regedit and extract their icons, using: "OSIcon.IconReader.GetFileTypeAndIcon();
".
Dictionary<string, string> _iconList = _iconList =
OSIcon.IconReader.GetFileTypeAndIcon();
foreach (KeyValuePair<string, string> list in _iconList)
Icon icon = OSIcon.IconReader.ExtractIconFromFile(_iconList[extension],
isLarge ? true : false);
Getting all icons on a file is quite simple, using: "ExtractIconsFromFile(path, isLarge);
".
filename = "C:\\Windows\\system32\\shell.dll";
Icon[] icons = IconReader.ExtractIconsFromFile(filename, true);
if(icons.Length == 0) return;
for (int i = 0; i < icons.Length; i++)
{
imageList.Images.Add(i.ToString(), icons[i]);
}
Sometimes, we need to display our files and have 100s of files of the same type, e.g., .php; you can reuse icons obtained from the first PHP file in that case. (Please see FileExplorer control)
IconProperties
class
IconsInfo
(stores icons information and indexes) Dictionary<IconReader.IconSize, IconInfo>
OSIcon.IconManager iconManager = new OSIcon.IconManager(true, true, true, true, true);
iconManager.AddFolder();
iconManager.AddComputerDrives();
iconManager.ImageList[IconReader.IconSize.Small].Images.Add(
":Up-icon:", Properties.Resources.Up_icon16x16);
iconManager.ImageList[IconReader.IconSize.Large].Images.Add(
":Up-icon:", Properties.Resources.Up_icon32x32);
if (OSIcon.Utils.IsXpOrAbove())
{
iconManager.ImageList[IconReader.IconSize.ExtraLarge].Images.Add(
":Up-icon:", Properties.Resources.Up_icon48x48);
}
if (OSIcon.Utils.IsVistaOrAbove())
{
iconManager.ImageList[IconReader.IconSize.Jumbo].Images.Add(
":Up-icon:", Properties.Resources.Up_icon256x256);
}
The class is initialized and ready to use; now, we create a function to show My Computer and the path contents:
void ShowMyComputer()
{
foreach (string drive in Directory.GetLogicalDrives())
{
OSIcon.IconManager.IconProperties iconProp =
OSIcon.iconManager.AddEx(drive, IconManager.IconSizeSupported);
var item = new ListViewItem(iconProp[IconSize.Small].DisplayName)
{
ImageIndex = iconProp[IconSize.Small].ItemIndex
};
item.SubItems.Add(string.Empty);
item.SubItems.Add(iconProp.IconsInfo[IconSize.Small].TypeName);
item.Tag = drive;
lvFileExplorer.Items.Add(item);
}
}
public void ShowPathContents(string path)
{
if (path == null)
return;
if (path == string.Empty)
{
ShowMyComputer();
return;
}
try
{
Cursor = Cursors.WaitCursor;
btnGoUp.Enabled = true;
RewindManager.Add(path);
btnGoBack.Enabled = RewindManager.CanPrevious;
btnGoForward.Enabled = RewindManager.CanForward;
lbSelected.Text = string.Empty;
CurrentPath = path;
lvFileExplorer.BeginUpdate();
lvFileExplorer.Items.Clear();
TotalFilesSize = 0;
TotalFolders = 0;
TotalFiles = 0;
foreach (var folder in Directory.EnumerateDirectories(path))
{
var dirInfo = new DirectoryInfo(folder);
if ((dirInfo.Attributes & FileAttributes.System) == FileAttributes.System)
continue;
var item = new ListViewItem(Path.GetFileName(folder)) { Tag = folder };
var iconProp = IconManager[IconManager.FolderClosed];
item.ImageIndex = iconProp[IconSize.Small].ItemIndex;
item.SubItems.Add(dirInfo.LastWriteTime.ToString(CultureInfo.CurrentCulture));
item.SubItems.Add(iconProp[IconSize.Small].TypeName);
item.SubItems.Add(string.Empty);
lvFileExplorer.Items.Add(item);
TotalFolders++;
}
foreach (var file in Directory.EnumerateFiles(path))
{
var fi = new FileInfo(file);
if ((fi.Attributes & FileAttributes.System) == FileAttributes.System)
continue;
var iconProp = IconManager.AddEx(fi.Extension, IconManager.IconsSizeSupported);
var item = new ListViewItem(fi.Name)
{
Tag = fi.FullName,
ImageIndex = iconProp[IconSize.Small].ItemIndex
};
item.SubItems.Add(fi.LastWriteTime.ToString(CultureInfo.CurrentCulture));
item.SubItems.Add(iconProp.IconsInfo[IconSize.Small].TypeName);
item.SubItems.Add(string.Format(" {0:##.##} {1}",
Utilities.FormatByteSize(fi.Length), Utilities.GetSizeNameFromBytes(fi.Length, false)));
lvFileExplorer.Items.Add(item);
TotalFilesSize += fi.Length;
TotalFiles++;
}
string textToSet = string.Empty;
if (TotalFolders > 0)
textToSet += string.Format("{0} {1}", TotalFolders,
Utilities.ConvertPlural(TotalFolders, "Folder"));
if (TotalFiles > 0)
{
if (textToSet != "Total:")
textToSet += " &";
textToSet += string.Format(" {0:##.##} {1} in {2} {3}",
Utilities.FormatByteSize(TotalFilesSize), Utilities.GetSizeNameFromBytes
(TotalFilesSize, false), TotalFiles, Utilities.ConvertPlural(TotalFiles, "File"));
}
if (textToSet == "Total:")
textToSet = "Empty";
tbAddress.Text = CurrentPath;
lbTotal.Text = textToSet;
}
catch (Exception ex)
{
MessageBox.Show(string.Format("Error on trying access: {0}\n\n{1}", path, ex.Message),
"Error", MessageBoxButtons.OK, MessageBoxIcon.Error);
}
finally
{
lvFileExplorer.EndUpdate();
Cursor = Cursors.Default;
RebuildNavigationHistory();
}
}
ListView
only has an "ImageList
" (should be 16 x 16px) and "LargeImageList
". If you want to show "ExtraLarge
" icons introduced in XP, and "Jumbo
" from Vista or above, you have to change "LargeImageList
" to the desired size, like:
private void ChangeListViewV(uint index)
{
if (index > 4)
{
switch (index)
{
case 5:
fileExplorerList.LargeImageList =
iconManager.ImageList[IconReader.IconSize.ExtraLarge];
break;
case 6:
fileExplorerList.LargeImageList =
iconManager.ImageList[IconReader.IconSize.Jumbo];
break;
}
fileExplorerList.View = View.LargeIcon;
return;
}
fileExplorerList.LargeImageList = iconManager.ImageList[IconReader.IconSize.Large];
fileExplorerList.View = (View)index;
}
How can you get rid of the added icons? It can be done like so:
iconManager.Remove(".txt", true);
iconManager.Remove(".wav", false);
iconManager.Remove(".exe", IconReader.IconSize.Small |
IconReader.IconSize.Jumbo, true);
You need not hard-code with the IconManager
class. Here is an example:
string name = ".dll";
IconProperties iconProp = iconManager.IconList[name];
if(!iconProp.IsValid(name, IconReader.IconSize.Small))
{
OSIcon.WinAPI.Shell32.SHFILEINFO shfi = new Shell32.SHFILEINFO();
iconManager.AddEx(name, IconReader.IconSize.Small, ref shfi);
}
if(!iconProp.IsValid(name, IconReader.IconSize.Jumbo))
{
OSIcon.WinAPI.Shell32.SHFILEINFO shfi = new Shell32.SHFILEINFO();
iconManager.AddEx(name, IconReader.IconSize.Jumbo, ref shfi);
}
IconProperties iconProp = iconManager.AddEx(name, IconReader.IconSize.Small | IconReader.IconSize.Jumbo);
V3.0
- Rewrite the code for better performance and usage
- Added a
FileExplorer
control that behaves like the Windows explorer - Added a strong key to the library
- Library is now compiled with .NET Framework 4.0
V2.0
- Added
About
class - Added better commentaries
- Added more samples
- Updated sample application to use new icon sizes
- Updated sample application to use the new library
IconProperties class
- Added the "
Remove
" function, to remove an icon from a specified size
- Supports multi sizes flags (
IconReader.IconSize.Small
| IconReader.IconSize.Large
) - Returns a
Dictionary<IconReader.IconSize, int>
with removed icons, where int
is the icon index on the ImageList
- Added three "
IsValid
" functions
bool IsValidEx(IconReader.IconSize size)
, same as "IsValid(IconReader.IconSize size)
", but also checks if the icon is not NULL
bool IsValid()
, checks if that instance contains an icon bool IsValid(IconReader.IconSize size)
, checks if an icon of a specified size exists in that instance
- Changed "
IconsInfo
" type from struct
to Dictionary<IconReader.IconSize, Shell32.SHFILEINFO>
- Changed "
IconsIndex
" type from struct
to Dictionary<IconReader.IconSize, int>
- Changed "
Icons
" type from struct
to Dictionary<IconReader.IconSize, Icon>
- Implemented a "
Tag
" object Disposable
class - "
IconProperties
" is now a class, was a struct
before
IconManager class
- Fixed the "
AddEx
" function to allow adding more icons of different sizes - Added commentaries
- Added two "
Remove
" functions, allows removing icons from the cache and from the ImageList
bool Remove(string path, bool removeIconFromList)
, removes all icons bool Remove(string path, IconReader.IconSize iconSize, bool removeIconFromList)
, removes icons of a specified size only
- Added
private
"Add
" function, common actions when adding icons to list (to remove redundancy) - Added "
IsValidEx
" function, same as "IsValid
", but returns the matched "IconProperties
"; otherwise returns a new instance - Added two new constructors:
public IconManager(bool createSmallIconList, bool createLargeIconList, bool createExtraLargeIconList, bool createJumboIconList)
public IconManager(bool createSmallIconList, bool createLargeIconList, bool createExtraLargeIconList, bool createJumboIconList, bool optimizeToOS)
- Replaced "
ImageListSmall
" and "ImageListLarge
" ImageLists
with "IImageList
" Dictionary<IconReader.IconSize, ImageList>
- Added "
IconSizeAllSupported
" readonly variable, contains all icon sizes supported by the current OS - Added "
IconManager.IconSizeAll
" constant, contains all icon sizes (Small | Large | ExtraLarge | Jumbo)
IconReader class
- Changed all functions that contain a "
IconReader.IconSize
" to support new icon sizes (ExtraLarge
, Jumbo
) - Added the "
ExtractIconFromResource
" function, extracts an icon by name from the assembly - Added the "
ExtractIconsFromFile
" function, extracts all icons from a file; returns "Icon[]
" - Added the "
ExtractIconFromFileEx
" function, identical to ExtractIconFromFile
, but supports bigger sizes and icon information - Added two new icon sizes to "
IconReader.IconSize
"
IconReader.Iconsize.ExtraLarge
(48x48 px.), XP or above supported IconReader.Iconsize.Jumbo
(256x256 px.), Vista or above supported
- Renamed function "
ExtractIcon
" to "ExtractIconFromFile
"
About class
- Added the "
ProjectAuthor
" constant, my name - Added the "
ProjecWWW
" constant, this page URL
V1.0.01
- Modified to correct egregious formatting and spelling errors - JSOP - 01/03/2010
V1.0