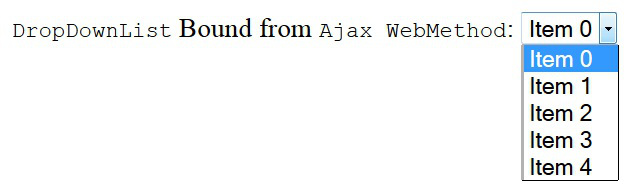
DropDownList Bound from Ajax WebMethod
We will take a look at one example of how to bind one DropDownList
using jQuery Ajax
and C# WebMethod
.
How?
Easy !!!
- Add a
WebMethod
to Code Behind page - Call that
WebMethod
from aspx
Page - Bind the
DropDownList
when call succeeds
Let’s See the Code
WebMethod
Here, we are just creating a Dummy DataTable
and converting it to XML
.
[System.Web.Services.WebMethod]
public static string GetDropDownItems(string tableName)
{
DataTable dt = new DataTable(tableName);
dt.Columns.Add("OptionValue");
dt.Columns.Add("OptionText");
dt.Rows.Add("0", "Item 0");
dt.Rows.Add("1", "Item 1");
dt.Rows.Add("2", "Item 2");
dt.Rows.Add("3", "Item 3");
dt.Rows.Add("4", "Item 4");
string result;
using (StringWriter sw = new StringWriter())
{
dt.WriteXml(sw);
result = sw.ToString();
}
return result;
}
JavaScript GetDropDownData Function
The following function would do a jQuery Ajax
call for the WebMethod
and bind the Data
to the DropDownList
.
function GetDropDownData() {
var ddlTestDropDownListXML = $('#ddlTestDropDownListXML');
var tableName = "someTableName";
$.ajax({
type: "POST",
url: "BindDropDownList.aspx/GetDropDownItems",
data: '{tableName: "' + tableName + '"}',
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function (response) {
$(response.d).find(tableName).each(function () {
var OptionValue = $(this).find('OptionValue').text();
var OptionText = $(this).find('OptionText').text();
var option = $("<option>" + OptionText + "</option>");
option.attr("value", OptionValue);
ddlTestDropDownListXML.append(option);
});
},
failure: function (response) {
alert(response.d);
}
});
}
Important: We are passing one parameter tableName
to the WebMethod
, which will be the Dummy Table Name and after conversion to XML
, it becomes parent of each Row. Refer to the following screenshot of returned XML Schema as seen inside Firebug Console.

XML returned from WebMethod
We can see that someTableName
is one one node containing the OptionText
and OptionValue
of a particular Item. These are actually Rows of the Dummy DataTable
before conversion to XML
.
Your Inputs !
Will be highly appreciated. Please feel free to comment. If you like the blog, share this among your friends and colleagues.
CodeProject
