Introduction
When we write code in C#, VB.NET, ASP.NET etc, we do use lot of inbuilt .NET framework classes to accomplish various tasks. When we have to include new classes, most we refer MSDN to find the exact class name and its namespace. Then we get to know about its methods, properties etc. This tool tries to accomplish most of the above said work without internet.
It takes data from Assembly reference files present in .NET framework folder and loads all data so that we can easily do a wild card search to search across classes, methods, properties,
Tool snapshot
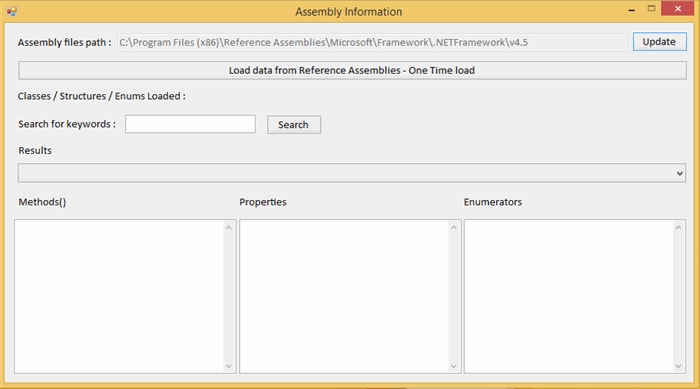
How to use the tool
-
The path of the assembly files is hardcoded to a 64 bit .NET 4.5 installer path
-
As needed, it can be updated per our wish. (UNC path also works)
-
Any non-existing path will be validated and only correct path is allowed
-
Once done, hit the big "Load data" button which loads all possible data to the tool memory and pops up a message box stating its complete
-
This is a one time process when tool is opened, and the memory is destroyed once tool is closed
-
Once loaded. you can see the count of data (classes, enums, structures etc) which are loaded
-
Then there is a search box which allows you to input the desired text we need to search in loaded data.
-
Once the search is hit, it loads every possible values into combo box present in the tool
-
As you choose the desired data in combo box automatically, all associated methods, properties and enum values are loaded in their respective result boxes
-
Also all the above are logged in a log file which will be named as AssemblyInformation<date>.log in the desired path defined in App.config file
Snapshot after a search

Using the code
The code uses System.Reflection namespace. Class Assembly and all its methods help to achieve this task. Important functions are below. Detailed explanation are given in comments and summary itself
1. Validate if DLL is a valid assembly or not. Not all DLLs are .NET assembly files
private bool isValidAssembly(string strFilePath)
{
try
{
Type[] asm = (Assembly.LoadFile(strFilePath)).GetTypes();
return true;
}
catch (Exception)
{
return false;
}
}
2. Load data from the assembly files. Load all data like namespaces, classes, enumerators from each DLL file. Say mscorlib.dll, System.dll etc
if (file.Substring(file.Length - 4).ToLower() == ".dll")
{
if (isValidAssembly(file))
{
Assembly asm = Assembly.LoadFile(file);
foreach (Type t in asm.GetTypes())
{
assemblyList.Add(t.AssemblyQualifiedName);
}
assemblyList = assemblyList.Distinct().ToList();
assemblyList.Sort();
}
else
{
assemblyfailedList.Add(file);
}
}
3. Once thats done, get methods, properties, enumerator details from Assembly functions like GetMethods(), GetProperties() etc.
Type myType1 = Type.GetType(strClassName);
foreach (MethodInfo methinfo in myType1.GetMethods())
{
if (!methinfo.Name.Contains("_"))
{
methodList.Add(methinfo.Name + "()");
}
}
methodList = methodList.Distinct().ToList();
methodList.Sort();
foreach (string strMethod in methodList)
{
methodname.AppendLine(strMethod);
}
txtMethods.Text += methodname.ToString();
4. Log all data to a log file for our future reference
private void logData(string strContent)
{
string strLogPath = ConfigurationManager.AppSettings["logpath"].ToString();
string strLogName = ConfigurationManager.AppSettings["logname"].ToString();
if (!strLogPath.Substring(strLogPath.Length - 1).Contains(@"\"))
{
strLogPath += @"\";
}
using (StreamWriter sr = new StreamWriter(strLogPath + strLogName + (int)DateTime.Now.DayOfWeek + ".log", true))
{
sr.WriteLine(strContent);
}
}
Points of Interest
Whenever we are writing code, we do need lot of references, which we normally search across MSDN with internet. With this tool, we dont need internet and it gives almost all related .NET assembly information. Also searches are saved for our future use, so that we dont need to search them again.
The idea came into mind, when I was working on a code, and my internet didnt work. It was very hard to find certain related class information, which is very easy for me to find out now.
References
Reflection : https://msdn.microsoft.com/en-us/library/ms173183.aspx
System.Reflection Namespace : https://msdn.microsoft.com/en-us/library/system.reflection(v=vs.110).aspx
History
1st revision - 02-Jan-2016. Trying to add more features, will update as I do the changes.