Introduction
I wrote for my web site a pretty control, based on a Partial View, which allows you to place vertical tabs on your web page easily with no JavaScript and minimum CSS.
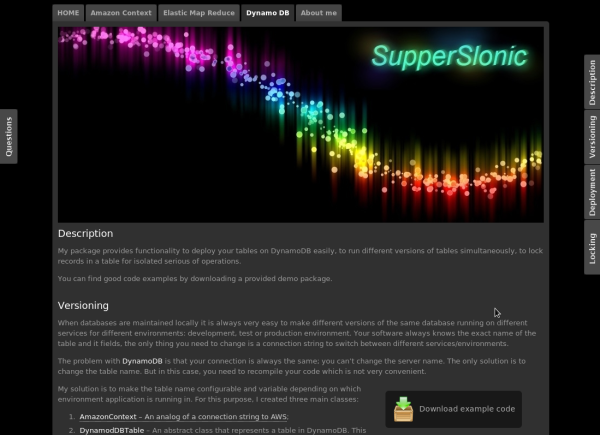
Using the code
Model
Everything begins with a model that describes a tab control. This model has the following properties:
Id
: A unique identifier of the element. Alignment
: Describes where the tab is located: on the left or on the right side of the screen.Top
: The offset that should be applied to a control from the top of the screen. Href
: Specifies where the browser should navigate on a click event. If not specified, will automatically generate an anchor based on the ID value,
for instance, “#description”. You can override it with any custom URL. -
TabImage
: For this tab control, image should be used as a background.
This property is resolved automatically by searching for an image with name equal to
the ID
property. For instance, “~/Content/img/description.png”. You can change this behavior,
for me it was just convenient.
public class TabWidgetModel
{
public TabWidgetModel(String id, Alignment alignment, String top)
{
this.Id = id;
this.Alignment = alignment;
this.Top = top;
this.Href = "#" + this.Id;
}
public TabWidgetModel(String id, Alignment alignment, String top, String href)
{
this.Id = id;
this.Alignment = alignment;
this.Top = top;
this.Href = href;
}
public String Id { get; set; }
public Alignment Alignment { get; set; }
public String Top { get; set; }
public String Href { get; set; }
public String TabImage
{
get { return "~/Content/img/" + this.Id + ".png"; }
}
}
Partial View
All common styles for tabs are stored in a CSS file. All styles particular for the specific tab are automatically generated in a view:
@model TabWidgetExample.Models.TabWidgetModel
<style>
body a#@Model.Id, body a#@Model.Id:link
{@Model.Alignment: 0;top: @this.Model.Top !important;margin-@this.Model.Alignment: -5px
!important;background-image: url(@Url.Content(this.Model.TabImage));}
body a#@this.Model.Id:hover
{ margin-@this.Model.Alignment: -3px !important; }
* html a#@this.Model.Id, * html a#@this.Model.Id:link
{ filter: progid:DXImageTransform.Microsoft.AlphaImageLoader(src='@Url.Content(this.Model.TabImage)'); }
</style>
<a id="@this.Model.Id" class="menu" href="@this.Model.Href"></a>
How to use
Populate a list of required tabs in a controller:
List<TabWidgetModel> model = new List<TabWidgetModel>();
model.Add(new TabWidgetModel("about", Alignment.Left, "100px", "http://supperslonic.com"));
model.Add(new TabWidgetModel("shop", Alignment.Right, "100px"));
model.Add(new TabWidgetModel("news", Alignment.Right, "201px"));
model.Add(new TabWidgetModel("search", Alignment.Right, "302px"));
return View(model);
Generate an HTML for the tabs in any place of you view using a Partial View:
@using TabWidgetExample.Models;
@model IList<TabWidgetModel>
@{
ViewBag.Title = "Index";
}
<html>
<head>
<title>Tab Widgets</title>
<link href="~/Content/css/tabWidget.css"
rel="stylesheet" type="text/css" />
</head>
<body>
...
@foreach (TabWidgetModel tabWidget in this.Model)
{ @Html.Partial("TabWidget", tabWidget) }
</body>
</html>