What is Prototype?
The prototype property allows you to add properties and methods to an object.
- Prototype is a property of function object.
- Only function objects can have prototype property.
Function
- Are objects.
- Returns value.
- Can return objects, including other functions.
- They have properties.
- They have methods.
- Can be copied, delete, augmented.
for example:
var foo = function() { };
Console.log(foo.prototype);
What is Object?
Anything created with the new keyword is an object.
for example:
new String("hi");
new Object();
new Array();
Person = function(name) {
this.name = name
}
Me = new Person("Imdadhusen");
Object will make your code much more cleaner and easy to maintain, It represents the context of your entire function or code block.
var timer = { start: 1000, stop: 2000 };
When you revisit the code after sometime, it will be bit difficult to remember or map why 2000 is used. But instead, if maintain an object and say 'stop', then you can be able to identify its significance.
Good Things Of Prototype
- Encourage modularity and re-usability
- Provide a consistent way to do (different) things
- Keep code to a minimum
- Make complexity easy
Augmenting Prototype
Adding constructor, properties and methods to prototype object.
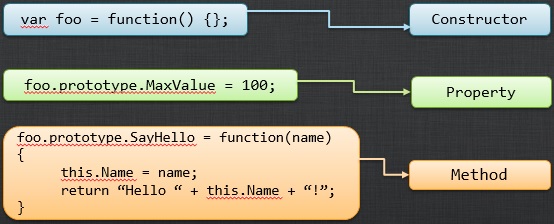
var myfoo = new foo();
console.log(myfoo.SayHello("Imdad"));
console.log(myfoo.Name);
Examples of Prototype
- String
- Number
- Date
- Array
- Function
- Using Pure JavaScript
- Using jQuery
String
The String.prototype property represents the prototype for the String constructor
Example:
alert("C:\www\scripts\views\Utils.js".getFileName());
String.prototype.getFileName = function(){
return this.match(/[^\\/]+\.[^\\/]+$/)[0];
}
alert("imdad{0}".isValidName());
String.prototype.isValidName = function() {
var regex = /^[a-zA-Z0-9 .\-_$@*!]{3,15}$/;
return regex.test(this);
}
alert("imdad@yahoo.com".isValidEmail());
String.prototype.isValidEmail = function() {
var regex = /^\w+@[a-zA-Z_]+?\.[a-zA-Z]{2,3}$/;
return regex.test(this);
}
Number
The Number.prototype property represents the prototype for the Number constructor
Example:
var start = 100;
var end = 200;
var value = 150;
if(value.isInRange(start,end))
alert('Value is in Range');
else
alert('Value is not in Range');
Number.prototype.isInRange = function(start,end){
this.Min = start||100;
this.Max = end||200;
return (this.Max >= this.valueOf() && this.Min <= this.valueOf());
}
Date
The Date.prototype property represents the prototype for the Date constructor
Example:
var dt = new Date();
alert(dt.shortDate());
alert(dt.fullDate());
Date.prototype.dayNames = ["Sunday", "Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday"];
Date.prototype.monthNames = ["January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"];
Date.prototype.fullDay = function() {
return this.dayNames[this.getDay()];
};
Date.prototype.shortDay = function() {
return this.fullDay().slice(0, 3);
};
Date.prototype.fullMonth = function() {
return this.monthNames[this.getMonth()];
};
Date.prototype.shortMonth = function() {
return this.fullMonth().slice(0, 3);
};
Date.prototype.shortYear = function() {
return this.getFullYear().toString().substr(2,2);
};
Date.prototype.shortDate = function() {
return this.shortDay() +', '+ this.getDate() +' '+ this.shortMonth() +' '+ this.shortYear();
}
Date.prototype.fullDate = function() {
return this.fullDay() +', '+ this.getDate() +' '+ this.fullMonth() +' '+ this.getFullYear()
}
Array
The Array.prototype property represents the prototype for the Array constructor
Example:
var users = new Array(
{ 'Country' : 'India', 'Code' : '+91'},
{ 'Country' : 'Afghanistan', 'Code' : '+93'},
{ 'Country' : 'Bhutan', 'Code' : '+975'},
{ 'Country' : 'Brazil', 'Code' : '+55'},
{ 'Country' : 'China', 'Code' : '+86'},
{ 'Country' : 'Egypt', 'Code' : '+20'},
{ 'Country' : 'Greece', 'Code' : '+30'}
);
users.renderList('#olUsersList');
users.removeByIndex(4);
users.renderList('#olUsersList');
var key = 'Country';
var val = 'Bhutan';
users.removeByObject(key, val);
users.renderList('#olUsersList');
Array.prototype.removeByIndex = function(index) {
this.splice(index, 1);
return this;
}
Array.prototype.removeByObject = function(key, val) {
var getUser = this.getObject(key, val);
if(getUser !== null)
this.splice(getUser.index, 1);
return this;
}
Array.prototype.getObject = function(key, val) {
for (var i=0; i < this.length; i++)
{
if (this[i][key] == val)
return { 'index': i , 'obj' : this[i] };
}
return null;
};
Array.prototype.renderList = function(ele) {
$(ele).empty();
this.forEach(function(item) {
$(ele).append('<li>'+>('+ item.Code +')');
});
}
</li>'+>
Function using jQuery
Using jquery we can extending our function object.
Example:
jQuery.fn.extend({
setScrollbar: function(height) {
return this.css({'overflow' : 'auto', 'height': height});
}
});
$(document).ready(function(){
$('#btnApplyjQueryScroll').click(function(){
$('#dvScoll_jQuery').setScrollbar(250);
});
});
Function using pure JavaScript
You can create your own custom control using pure JavaScript.
Example:
function myScroll(ele){
this.element = document.getElementById(ele);
}
myScroll.prototype.setScrollbar = function(height){
this.element.style.height = height + 'px';
this.element.style.overflow = 'auto';
}
$(document).ready(function(){
$('#btnApplyJSScroll').click(function(){
var t = new myScroll('dvScoll_JS');
t.setScrollbar(150);
});
});
I have shown above all the examples are beginner level developer. They will get more clear idea about how prototype works for each datatype. If you would like to know about more please let me know.